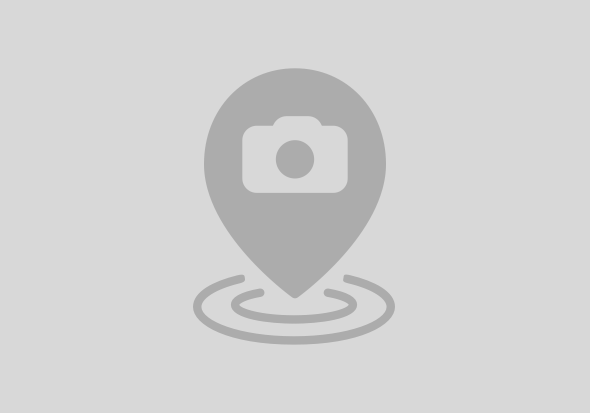
This blog post aims at explaining the use of Authorization Management API for HCP applications particularly Fiori Launchpad. Since there is no proper explanation on how to use the API anywhere properly, this post will simplify and help you to implement quickly. But you can always go through the documentation.Here are the links :
This API is used to assign Users to particular custom made Roles.
To understand this , please go through this link: Managing Roles
By assigning users to particular roles, we can segregate users and display their respective Tiles on launchpad based on their roles. For example, User1 is assigned to Role A and User2 is assigned to Role B. So Role A will be showing tiles Tile1, Tile2 and Tile3 while Role B will be showing tiles Tile4, Tile5 and Tile6. This helps in securing and separating different types of users.
So the authorization management REST API provides functionality to manage roles and their assignments to users. The API is protected with OAuth 2.0. To understand OAuth 2.0 better visit this link OAuth simplified .
In order to create custom roles , go to the HCP cockpit, select subscriptions and under the java section click on sandbox which is our Fiori Launchpad Java app. Once we click on this, we get the Roles section. Here you can create your custom roles. For example roles Carrier, Shipper and Admin have been created here. So once you create roles here (in HCP) for the sandbox application, it automatically gets mapped to the Roles in your Fiori Configuration Cockpit. And then you can create your groups there and assign the roles to respective groups and catalogs.
Once the roles have been created, all we need to do is assign users to the particular roles dynamically. So now the Authorization Management API comes into the picture. In order to use the API we need to get the access token using the Client ID and Client Secret.
Once the Client Id and secret is obtained , note them down somewhere because we can't Copy-Paste it 😛 Now use the postman client to get the Access Token. So the URL to POST would be https://api.int.sap.hana.ondemand.com/oauth2/apitoken/v1 . Choose Authorization as Basic Auth and enter Client Id and Secret for Username and Password respectively. So once you POST this , you will be getting an access token.
So once we have obtained the access token we need to make PUT requests in order to assign an User to a Role. So the URL to PUT would be https://api.int.sap.hana.ondemand.com/authorization/v1/accounts/we5bd8b5a/users/roles?userId=emailid . Here the emailid is the email id of the user you want to add to the particular role. In the Headers , enter Authorization and give value as Bearer <<accesstokenvalue>> and then enter Content-Type and value as application/json . So once the headers are entered, in the body choose the type raw and this should be the value
{
"roles": [
{
"name": "Admin",
"applicationName": "sandbox",
"providerAccount": "portal"
}
]
}
Here name is the rolename which would be Admin or Shipper or Carrier in my case.
So now go back to HCP cockpit and click on Admin Role for sandbox app, and you will see that the user with emailid has been added successfully.
Apart from assigning users to particular roles , we can also get users for a particular role or groups , and get the roles assigned to a particular user. So these would be the GET URLS, and we need to add only the Authorization Header with Bearer and access token value.
package com.cce.AuthorizationManagement;
import java.io.IOException;
import javax.naming.NamingException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.gson.Gson;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.sap.cloud.security.password.PasswordStorageException;
import com.cce.AuthorizationManagement.Handler.AuthApiHandler;
import com.cce.AuthorizationManagement.Handler.OauthHandler;
import com.cce.keystore.passwordKeyStore;
import com.cce.utils.Util;
public class AuthorizationApiUserManagementWs extends HttpServlet {
private static final long serialVersionUID = 1L;
private Gson gson = new Gson();
AuthApiHandler authApiHandler = new AuthApiHandler();
private static Logger logger = LoggerFactory.getLogger(AuthorizationApiUserManagementWs.class);
public AuthorizationApiUserManagementWs() {
super();
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
logger.debug("Entering AuthorizationApiUserManagementWs.doGet Method");
String oAuthResponse, userList, accessToken;
JsonElement element;
JsonObject jsonObj;
char[] pass = null;
String roleName = request.getParameter("roleName");
String groupName = request.getParameter("groupName");
try {
if ((pass = passwordKeyStore.getInstance().getPassword("accessToken")) != null) {
try {
logger.debug("Accessing Key From KeyStore");
String passString = new String(pass);
if (passString !=null){
response.getWriter().append("pass :"+ passString );
}
if (roleName == null && groupName == null) {
logger.error("roleName / groupName Parameter Not Provided");
response.setStatus(400);
response.getWriter().append("roleName/groupName Parameter Missing");
return;
}
if(roleName!= null){
userList = authApiHandler.getUsers(passString, request.getParameter("roleName"));
response.getWriter().append(userList);
return;
}
if(groupName!= null){
userList = authApiHandler.getGroups(passString, request.getParameter("groupName"));
response.getWriter().append(userList);
return;
}
} catch (IOException e) {
logger.debug("Token in PasswordStore Expired");
}
}
logger.debug("Accessing Key From OAuth Api");
try {
oAuthResponse = OauthHandler.oauthApiCaller();
} catch (IOException e) {
Util.exceptionResponseHandler("Exception occured when oAuthAPI Called", 500, e, response);
return;
}
element = gson.fromJson(oAuthResponse, JsonElement.class);
jsonObj = element.getAsJsonObject();
accessToken = jsonObj.get("access_token").getAsString();
passwordKeyStore.getInstance().setPassword("accessToken", accessToken.toCharArray());
try {
userList = authApiHandler.getUsers(accessToken, (roleName != null) ? roleName : "");
response.getWriter().append(userList);
return;
} catch (IOException e) {
Util.exceptionResponseHandler("authApiHandler.getUsers IOException Error", 500, e, response);
return;
}
} catch (PasswordStorageException e) {
Util.exceptionResponseHandler("Internal Error", 500, e, response);
} catch (NamingException e) {
Util.exceptionResponseHandler("Internal Error", 500, e, response);
}
logger.debug("Exiting AuthorizationApiUserManagementWs.doGet Method");
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String oAuthResponse, recc, accessToken;
String body = Util.getHttpRequestAsString(request);
AuthApiHandler aah = new AuthApiHandler();
JsonElement element;
JsonObject jsonObj;
char[] pass = null;
String roleName = request.getParameter("roleName");
try {
if ((pass = passwordKeyStore.getInstance().getPassword("accessToken")) != null) {
try {
logger.debug("Accessing Key From KeyStore");
String passString = new String(pass);
if (roleName == null) {
logger.error("roleName Parameter Not Provided");
response.setStatus(400);
response.getWriter().append("roleName Parameter Missing");
return;
}
authApiHandler.addUsers(passString, (roleName != null) ? roleName : "", body);
return;
} catch (IOException e) {
logger.debug("Token Expired");
}
}
logger.debug("Accessing Key From OAuth Api");
try {
oAuthResponse = OauthHandler.oauthApiCaller();
} catch (IOException e) {
Util.exceptionResponseHandler("Invalid Token", 401, e, response);
return;
}
element = gson.fromJson(oAuthResponse, JsonElement.class);
jsonObj = element.getAsJsonObject();
accessToken = jsonObj.get("access_token").getAsString();
passwordKeyStore.getInstance().setPassword("accessToken", accessToken.toCharArray());
try {
recc = aah.addUsers(accessToken, request.getParameter("roleName"), body);
response.getWriter().append(recc);
return;
} catch (IOException e) {
Util.exceptionResponseHandler("Invalid Token addUsers", 401, e, response);
return;
}
} catch (PasswordStorageException e) {
Util.exceptionResponseHandler("Internal Error", 500, e, response);
} catch (NamingException e) {
Util.exceptionResponseHandler("Internal Error", 500, e, response);
}
}
@Override
protected void doPut(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// TODO Auto-generated method stub
String oAuthResponse, recc, accessToken;
String body = Util.getHttpRequestAsString(req);
AuthApiHandler aah = new AuthApiHandler();
JsonElement element;
JsonObject jsonObj;
char[] pass = null;
String userId = req.getParameter("userId");
try {
if ((pass = passwordKeyStore.getInstance().getPassword("accessToken")) != null) {
try {
logger.debug("Accessing Key From KeyStore");
String passString = new String(pass);
if (userId == null) {
logger.error("userId Parameter Not Provided");
resp.setStatus(400);
resp.getWriter().append("userId Parameter Missing");
return;
}
authApiHandler.addRoles(passString, (userId != null) ? userId : "", body);
return;
} catch (IOException e) {
logger.debug("Token Expired");
}
}
logger.debug("Accessing Key From OAuth Api");
try {
oAuthResponse = OauthHandler.oauthApiCaller();
} catch (IOException e) {
Util.exceptionResponseHandler("Invalid Token", 401, e, resp);
return;
}
element = gson.fromJson(oAuthResponse, JsonElement.class);
jsonObj = element.getAsJsonObject();
accessToken = jsonObj.get("access_token").getAsString();
passwordKeyStore.getInstance().setPassword("accessToken", accessToken.toCharArray());
try {
recc = aah.addUsers(accessToken, req.getParameter("userId"), body);
resp.getWriter().append(recc);
return;
} catch (IOException e) {
Util.exceptionResponseHandler("Invalid Token addRoles", 401, e, resp);
return;
}
} catch (PasswordStorageException e) {
Util.exceptionResponseHandler("Internal Error", 500, e, resp);
} catch (NamingException e) {
Util.exceptionResponseHandler("Internal Error", 500, e, resp);
}
}
}
package com.cce.AuthorizationManagement.Handler;
import java.io.IOException;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.cce.utils.HttpClientUtil;
import com.cce.utils.ReadProperties;
public class AuthApiHandler{
private static String landscape = ReadProperties.getInstance().getValue("LandscapeHost");
private static String appName = ReadProperties.getInstance().getValue("AppName");
private static String accountName = ReadProperties.getInstance().getValue("AccountName");
private static Logger logger = LoggerFactory.getLogger(AuthApiHandler.class);
public String getUsers(String oAuthToken, String role) throws IOException{
logger.debug("Entering AuthApiHandler.getUsers Method");
HttpClientUtil client = new HttpClientUtil();
String url = new StringBuilder("https://api.int.sap.hana.ondemand.com/authorization/v1/accounts/we5bd8b5a/apps/sandbox/roles/users?roleName=Admin&providerAccount=portal").toString(); //("https://api.")
//.append(landscape)
//.append("/authorization/v1/accounts/")
//.append(accountName)
//.append("/apps/")
//.append(appName)
//.append("/roles/users?roleName=")
//.append(role).toString();
Map<String,String> response = client.getData(url ,"Bearer "+ oAuthToken);
if(!response.get("status").equals("200")){
logger.error("HTTP getUsers GET Request at AuthApiHandler failed");
throw new IOException("HTTP getUsers GET Request at AuthApiHandler failed");
}
logger.debug("Exiting AuthApiHandler.getUsers Method");
return response.get("body");
}
public String getGroups(String oAuthToken, String group) throws IOException{
logger.debug("Entering AuthApiHandler.getUsers Method");
HttpClientUtil client = new HttpClientUtil();
String url = new StringBuilder("https://api.")
.append(landscape)
.append("/authorization/v1/accounts/")
.append(accountName)
//.append("/apps/")
//.append(appName)
//.append("/roles/users?roleName=")
.append("/groups/users?groupName=") //carrier_group").toString();
.append(group).toString();
Map<String,String> response = client.getData(url ,"Bearer "+ oAuthToken);
if(!response.get("status").equals("200")){
logger.error("HTTP getGroups GET Request at AuthApiHandler failed");
throw new IOException("HTTP getGroups GET Request at AuthApiHandler failed");
}
logger.debug("Exiting AuthApiHandler.getGroups Method");
return response.get("body");
}
public String addUsers(String oAuthToken, String role, String users) throws IOException{
logger.debug("Entering AuthApiHandler.addUsers Method");
HttpClientUtil client = new HttpClientUtil();
String url = new StringBuilder("https://api.")
.append(landscape)
.append("/authorization/v1/accounts/")
.append(accountName)
.append("/apps/")
.append(appName)
.append("/roles/users?roleName=")
.append(role).toString();
Map<String,String> response = client.putData(url ,users,"Bearer "+ oAuthToken);
if(!response.get("status").equals("201")){
logger.error("HTTP addUsers PUT Request at AuthApiHandler failed");
throw new IOException("HTTP addUsers PUT Request at AuthApiHandler failed");
}
logger.debug("Exiting AuthApiHandler.addUsers Method");
return response.get("body");
}
public String addRoles(String oAuthToken, String userId, String roles) throws IOException{
logger.debug("Entering AuthApiHandler.addUsers Method");
HttpClientUtil client = new HttpClientUtil();
String url = new StringBuilder("https://api.")
.append(landscape)
.append("/authorization/v1/accounts/")
.append(accountName)
//.append("/apps/")
//.append(appName)
.append("/users/roles?userId=")
.append(userId).toString();
Map<String,String> response = client.putData(url ,roles,"Bearer "+ oAuthToken);
if(!response.get("status").equals("201")){
logger.error("HTTP addRoles PUT Request at AuthApiHandler failed");
throw new IOException("HTTP addRoles PUT Request at AuthApiHandler failed");
}
logger.debug("Exiting AuthApiHandler.addRoles Method");
return response.get("body");
}
public String deleteUsers(String oAuthToken, String role, String users) throws IOException{
logger.debug("Entering AuthApiHandler.deleteUsers Method");
HttpClientUtil client = new HttpClientUtil();
String url = new StringBuilder("https://api.")
.append(landscape)
.append("/authorization/v1/accounts/")
.append(accountName)
.append("/apps/")
.append(appName)
.append("/roles/users?roleName=")
.append(role).toString();
Map<String,String> response = client.deleteData(url ,"Bearer "+ oAuthToken);
if(!response.get("status").equals("200")){
logger.error("HTTP deleteUsers DELETE Request at AuthApiHandler failed");
throw new IOException("HTTP deleteUsers DELETE Request at AuthApiHandler failed");
}
logger.debug("Exiting AuthApiHandler.deleteUsers Method");
return response.get("body");
}
public String getRoles(String oAuthToken) throws IOException{
logger.debug("Entering AuthApiHandler.getRoles Method");
HttpClientUtil client = new HttpClientUtil();
String url = new StringBuilder("https://api.")
.append(landscape)
.append("/authorization/v1/accounts/")
.append(accountName)
.append("/apps/")
.append(appName)
.append("/roles")
.toString();
Map<String,String> response = client.getData(url ,"Bearer "+ oAuthToken);
if(!response.get("status").equals("200")){
logger.error("HTTP addUsers PUT Request at AuthApiHandler failed");
throw new IOException("HTTP addUsers PUT Request at AuthApiHandler failed");
}
logger.debug("Exiting AuthApiHandler.addUsers Method");
return response.get("body");
}
}
package com.cce.AuthorizationManagement.Handler;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.Map;
import javax.xml.bind.DatatypeConverter;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.cce.utils.HttpClientUtil;
import com.cce.utils.Util;
public class OauthHandler {
// static HttpResponse oAuthResponse;
private static final String CLIENTID = "User";
private static final String CLIENT_SECRET = "Password";
private static final Logger LOGGER = LoggerFactory.getLogger(OauthHandler.class);
public static String oauthApiCaller() throws IOException {
Map<String, String> oAuthResponse;
HttpClientUtil client = new HttpClientUtil();
Map<String, String> oAuthDetails = Util.getOAuthDetails();
if (oAuthDetails == null) {
throw new IOException();
}
String clientId = oAuthDetails.get(OauthHandler.CLIENTID);
String clientSecret = oAuthDetails.get(OauthHandler.CLIENT_SECRET);
String oAuthDestination = oAuthDetails.get("URL");
String combinedKeySet = new StringBuilder(clientId).append(":").append(clientSecret).toString();
String base64String = DatatypeConverter.printBase64Binary(combinedKeySet.getBytes(StandardCharsets.UTF_8));
oAuthResponse = client.postData(oAuthDestination, "", "Basic " + base64String);
if (!oAuthResponse.get("status").equals("200")) {
LOGGER.error("HTTP Request at OauthHandler failed");
throw new IOException("HTTP Request at OauthHandler failed");
}
return oAuthResponse.get("body");
}
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
23 | |
14 | |
13 | |
13 | |
11 | |
10 | |
9 | |
9 | |
8 | |
8 |