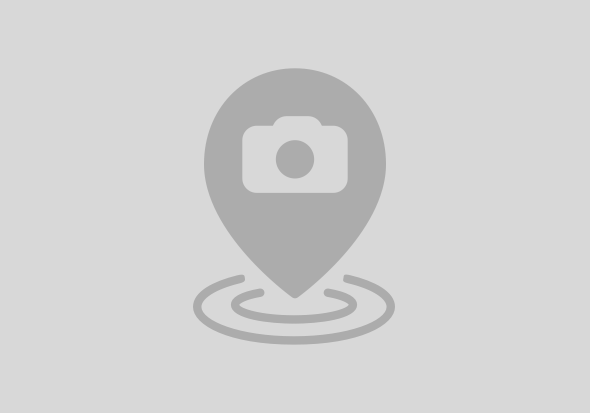
ThreadContext
has seen major changes in the SAP Cloud SDK.ThreadContext
attached.ThreadContext
.ThreadContext
.@RestController
public class HelloWorldController
{
@GetMapping( "/hello" )
public String getHello()
{
final ThreadContext currentContext = ThreadContextAccessor.getCurrentContext();
database.store(currentTenant);// long-running operation
return "Hello";
}
}
ThreadContext
.@Async
annotated method:@RestController
public class HelloWorldController
{
@Autowired
private DatabaseAccess databaseAccess;
@GetMapping( "/hello" )
public String getHello()
{
databaseAccess.store();
return "Hello";
}
}
@Async
method must be public in another class to be executed in a separate thread:@EnableAsync
@Configuration
public class DatabaseAccess
{
@Async
public String store()
{
final ThreadContext currentContext = ThreadContextAccessor.getCurrentContext();// doesn't work
database.store(currentTenant);// long-running operation
return "success";
}
}
ThreadContextExecutor
to propagate the ThreadContext
to the new thread:@RestController
public class HelloWorldController
{
@Autowired
private DatabaseAccess databaseAccess;
@GetMapping( "/hello" )
public String getHello()
{
final ThreadContextExecutor executor = new ThreadContextExecutor();
databaseAccess.store(executor);
return "Hello";
}
}
@EnableAsync
@Configuration
public class DatabaseAccess
{
@Async
public String store( ThreadContextExecutor executor )
{
return executor.execute(() -> {
final ThreadContext currentContext = ThreadContextAccessor.getCurrentContext();// unreliable
database.store(currentTenant);// long-running operation
return "success";
});
}
}
ThreadContext
will get removed when the servlet is finished.ThreadContextExecutors
:Future runningTask = ThreadContextExecutors.submit(() -> operation());
@Async
annotation like so:@RestController
public class HelloWorldController
{
@Autowired
private DatabaseAccess databaseAccess;
@GetMapping( "/hello" )
public String getHello()
{
databaseAccess.store();
return "Hello";
}
}
@Configuration
public class DatabaseAccess
{
@Async
public void store()
{
database.store(TenantAccessor.getCurrentTenant());// long-running operation
}
}
@Configuration
all methods annotated with @Async
will have the ThreadContext
available:@EnableAsync
@Configuration
public class AsynchronousConfiguration implements AsyncConfigurer
{
@Override
public Executor getAsyncExecutor()
{
return ThreadContextExecutors.getExecutor();
}
}
scp-cf-spring
.@Async
functionality here.ThreadContextExecutors
class leverages a single ThreadContextExecutorService
instance that can be configured.ThreadContextExecutorService
, for example to use a different thread pool, via:ThreadContextExecutorService executor = DefaultThreadContextExecutorService
.of(Executors.newFixedThreadPool(3));
// use it directly:
executor.submit(myTask);
// or set it to be used by the static ThreadContextExecutors API:
ThreadContextExecutors.setExecutor(executor);
ThreadContextExecutors.submit(myTask);
RequestContext
.cds-integration-cloud-sdk
dependency to propagate the context out of the box when using our APIs.ThreadContextExecutors
allows you to run tasks on different threads with the ThreadContext
attached.You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
17 | |
17 | |
12 | |
11 | |
9 | |
9 | |
8 | |
8 | |
7 | |
7 |