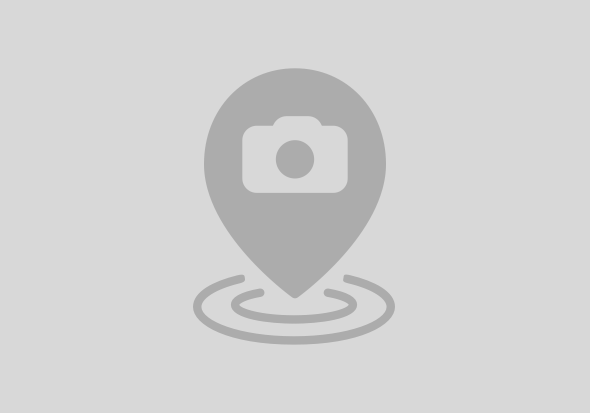
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-starter-webmvc-ui</artifactId>
<version>2.2.0</version>
</dependency>
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/swagger/**")
.addResourceLocations("classpath:/swagger/");
}
@Operation(summary = "Post Data to monitoring")
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Data has been consumed successfully", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = IntegrationExecution.class)) }),
@ApiResponse(responseCode = "500", description = "TaskRejectException", content = @Content) })
@Bean
public OpenAPI customOpenAPI() {
return new OpenAPI().info(new Info().title("MIS")
.version("1.0.0"))
.components(new Components().addSecuritySchemes("Authorization",
new SecurityScheme().type(SecurityScheme.Type.APIKEY)
.in(SecurityScheme.In.HEADER)
.name("Authorization")))
.addSecurityItem(new SecurityRequirement().addList("Authorization"));
}
<plugin>
<groupId>io.swagger.codegen.v3</groupId>
<artifactId>swagger-codegen-maven-plugin</artifactId>
<version>3.0.33</version>
<executions>
<execution>
<goals>
<goal>generate</goal>
</goals>
<configuration>
<inputSpec>${project.basedir}/src/main/resources/swagger/openapiInput.json</inputSpec>
<!-- Use 'openapi-yaml' to get resolved YAML or 'openapi' to get resolved JSON -->
<language>openapi</language>
<!-- Default is ${project.build.directory}/generated-sources/swagger -->
<output>${project.basedir}/src/main/resources/swagger/</output>
<configOptions>
<!-- Default output file name is 'openapi.yaml' or 'openapi.json' -->
<outputFile>openapi.json</outputFile>
</configOptions>
</configuration>
</execution>
</executions>
</plugin>
{
"openapi": "3.0.2",
"info": {
"title": "Swagger-CAP Java",
"description": "Swagger implementation for CAP JAVA MVC",
"termsOfService": "http://swagger.io/terms/",
"contact": {
"email": "apiteam@swagger.io"
},
"license": {
"name": "Apache 2.0",
"url": "http://www.apache.org/licenses/LICENSE-2.0.html"
},
"version": "1.0.11"
},
"servers": [
{
"url": "http://localhost:8080"
}
],
"tags": [
{
"name": "postData",
"description": "Post data to sample app"
}
],
"paths": {
"/webhook": {
"post": {
"tags": [
"postData"
],
"summary": "Add post data to sample app",
"description": "Add post data to sample app",
"operationId": "postData",
"requestBody": {
"description": "Create a new post record",
"content": {
"application/json": {
"schema": {
"$ref": "#/components/schemas/RequestBody"
}
}
},
"required": true
},
"responses": {
"200": {
"description": "Successful operation",
"content": {
"application/json": {
"schema": {
"$ref": "#/components/schemas/RequestBody"
}
}
}
},
"500": {
"description": "TaskRejectException"
}
},
"security": [
{
"ApiKey_auth": [
"write:postData"
],
"oauth2": [
"write:postData"
]
}
]
}
}
},
"components": {
"schemas": {
"RequestBody": {
"type": "object",
"properties": {
"keyID": {
"type": "string",
"format": "UUID"
},
"key_code": {
"type": "string",
"example": "S- Scheduled/ M- Manual"
},
"status_code": {
"type": "string",
"example": "C- Completed/ D- Errors/ F- Failed"
},
"startRunTimestamp": {
"type": "date-time"
},
"logs": {
"$ref": "#/components/schemas/Logs"
},
"errors": {
"$ref": "#/components/schemas/Errors"
},
"reports": {
"$ref": "#/components/schemas/reports"
}
}
},
"reports": {
"type": "object",
"example": "UUID"
},
"Logs": {
"type": "object",
"properties": {
"logTimestamp": {
"type": "date-time"
},
"logMessage": {
"type": "string"
},
"logLevel_code": {
"type": "string",
"example": "I- Info/ W- Warning/ E- Error"
}
}
},
"Errors": {
"type": "object",
"properties": {
"errorType_ID": {
"type": "string",
"example": "D - Data Error/ I - Integration Error"
},
"errorCode": {
"type": "integer"
},
"errorDescription": {
"type": "string"
},
"errorCategory_ID": {
"type": "string",
"example": "SCRIPT_RUNTIME_ERROR"
},
"errorTimestamp": {
"type": "date-time"
},
"reportID": {
"type": "string",
"format": "UUID"
}
}
}
},
"securitySchemes": {
"ApiKey_auth": {
"type": "apiKey",
"name": "Authorization",
"in": "header"
},
"oauth": {
"type": "oauth2",
"flows": {
"clientCredentials": {
"tokenUrl": <URL>,
"scopes": {
"write:postData": "add records in sample app"
}
}
}
}
}
}
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
24 | |
9 | |
8 | |
7 | |
7 | |
6 | |
6 | |
6 | |
6 | |
6 |