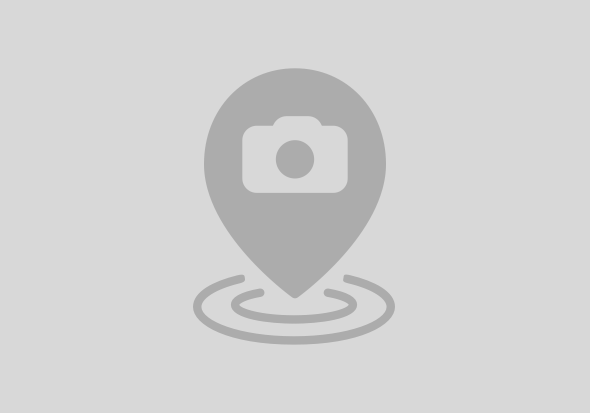
Category | Class | Description |
Host support | HostFactory Host | A Host is equivalent to a new job in the Promotion Management tool. |
LCM Archive Support | LcmArchiveFactory LcmArchive | Used to set the import or export Lcm Archive filename and path depending on the scenario |
Scenario Support | ImportScenario ExportScenario PromoteScenario ScenarioOptions | Defines the scenario to be created and run by the Host. You can run, schedule or test promote (dryRun) the scenario. The ScenarioOptions class allows setting security, comment and rollback options. |
Resource Selection | ObjectBrowser ObjectMatcher | Similar to the Add Objects window, these classes let you add or remove objects as InfoObjects, CUIDs or from a query and compute dependencies. |
Execution Support | RunResult SchedulingStatus | Allows for tracking the status of a job while executing |
User Feedback | Logger ILoggerOutput IProgressMonitor AbstractProgressMonitor. | This is not part of the UI but these classes provide the ability to log and follow progress of a job. |
public class ExportToLcmbiar {
// Export Scenario for Live to LCMBiar
public static void main(String[] args) throws SDKException, LCMException {
// log on to CMS that will run the job
IEnterpriseSession mySession;
ISessionMgr sessionManager = CrystalEnterprise.getSessionMgr();
mySession = sessionManager.logon( userName, password, myCMS, authType);
mySession.setLocale(Locale.ENGLISH);
// check that logged on user has Admin Rights
boolean isAdmin = com.businessobjects.sdk.lcm.SDKUtils.isConnectedAsAdmin(mySession);
System.out.println("user is Admin: " + isAdmin);
//get all objects from the folder with ID 1234
String qry = "SELECT SI_CUID from CI_INFOOBJECTS where SI_PARENTID=1234";
//Create a new LCM Job to export to lcmbiar file
Host host = HostFactory.newInstance(mySession);
ExportScenario myScenario = host.newExportScenario("LCMSDK_ExportTest");
//Log onto Source CMS
IEnterpriseSession sourceSession = sessionManager.logon(srcUserName, srcPassword, srcCMS, authType);
//set the export destination for the LCMBiar file
LcmArchive myExport = LcmArchiveFactory.newInstance("c:\\test\\SDKExport.lcmbiar");
myScenario.setDestination(myExport);
//Add objects to be exported
ObjectBrowser oBrowser = myScenario.setSource(sourceSession);
oBrowser.addQuery(qry);
oBrowser.computeDependencies();
for (Set<IInfoObject> dependencies :oBrowser.getDependencies().values())
{
oBrowser.addObjects(dependencies);
}
//Run the scenario and release the host
myScenario.run();
host.release();
//log off
sourceSession.logoff();
mySession.logoff();
System.out.println("Session Logged off");
}
public class ImportFromLcmbiar {
//Import Scenario from lcmbiar file to live CMS
public static void main(String[] args) throws SDKException, LCMException {
// TODO Auto-generated method stub
// log on to host CMS
IEnterpriseSession mySession;
ISessionMgr sessionManager = CrystalEnterprise.getSessionMgr();
mySession = sessionManager.logon( userName, password, myCMS, authType);
mySession.setLocale(Locale.ENGLISH);
// check that logged on user has Admin Rights
boolean isAdmin = com.businessobjects.sdk.lcm.SDKUtils.isConnectedAsAdmin(mySession);
System.out.println("user is Admin: " + isAdmin);
//Create a new LCM Job to import lcmbiar file
Host host = HostFactory.newInstance(mySession);
ImportScenario myScenario = host.newImportScenario("LCMSDK_ImportTest");
//load source file for import job.
String importPath = "c:\\test\\SDKimport.lcmbiar";
LcmArchive importFile = LcmArchiveFactory.newInstance(importPath);
importFile.getFile();
myScenario.setSource(importFile);
//Log onto Destination CMS
IEnterpriseSession destinationSession = sessionManager.logon(userName, password, destinationCMS, authType);
myScenario.setDestination(destinationSession);
myScenario.run();
host.release();
//Log off all sessions
destinationSession.logoff();
mySession.logoff();
System.out.println("Session Logged off");
}
public class promoteLiveToLive {
static String myCMS = "localhost";
static String sourceCMS = "192.168.1.65";
static String destinationCMS = "localhost";
static String authType = "secEnterprise";
static String userName = "Administrator";
static String password = "Secret123";
public static void main(String[] args) throws SDKException, LCMException {
// TODO Auto-generated method stub
// log on to source CMS
IEnterpriseSession mySession;
ISessionMgr sessionManager = CrystalEnterprise.getSessionMgr();
mySession = sessionManager.logon( userName, password, myCMS, authType);
mySession.setLocale(Locale.ENGLISH);
// check that logged on user has Admin Rights
boolean isAdmin = com.businessobjects.sdk.lcm.SDKUtils.isConnectedAsAdmin(mySession);
System.out.println("user is Admin: " + isAdmin);
//Create a new LCM Job to promote from an older system
Host host = HostFactory.newInstance(mySession);
PromoteScenario myScenario = host.newPromoteScenario("LCMSDK_PromoteTest");
//Log onto Source CMS
IEnterpriseSession sourceSession = sessionManager.logon(userName, password, sourceCMS, authType);
//contents of samples folder on older BI4.1SP5 system
String qry = "SELECT SI_CUID from CI_INFOOBJECTS where SI_PARENTID=186681";
//Add objects to be exported
ObjectBrowser oBrowser = myScenario.setSource(sourceSession);
oBrowser.addQuery(qry);
oBrowser.computeDependencies();
for (Set<IInfoObject> dependencies :oBrowser.getDependencies().values())
{
oBrowser.addObjects(dependencies);
}
//Log onto Destination CMS
IEnterpriseSession destinationSession = sessionManager.logon(userName, password, destinationCMS, authType);
myScenario.setDestination(destinationSession);
myScenario.run();
host.release();
//log off all sessions
mySession.logoff();
sourceSession.logoff();
destinationSession.logoff();
System.out.println("Session Logged off");
}
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
23 | |
14 | |
13 | |
13 | |
11 | |
10 | |
9 | |
9 | |
8 | |
8 |