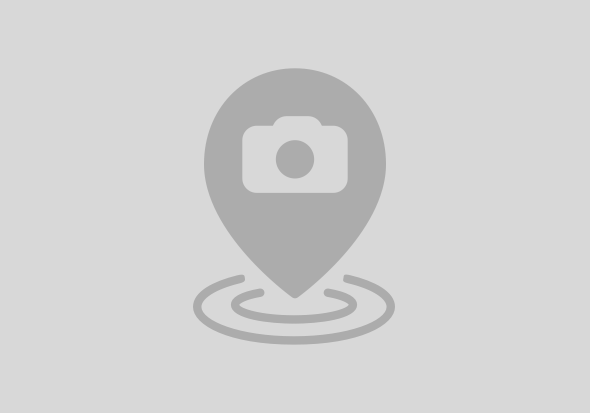
let oModel = this.getView().getModel();
let oBindList = oModel.bindList("/Books");
oBindList.create({
title: "Test Book",
stock: 100,
description: "Test Description"
});
handleRead: function(oEvent){
let oModel = this.getView().getModel();
let oBindList = oModel.bindList("/Books");
oBindList.requestContexts().then(function (aContexts) {
aContexts.forEach(oContext => {
console.log(oContext.getObject());
});
});
}
handleRead: function(oEvent){
let oModel = this.getView().getModel();
let bookID = oEvent.getSource().getBindingContext().getObject().ID;
let aFilter = new sap.ui.model.Filter("ID", sap.ui.model.FilterOperator.EQ, bookID);
oModel.bindList("/Books", undefined, undefined, aFilter, {
$expand: "author($expand=book)"
}).requestContexts().then(function (aContexts) {
aContexts.forEach(oContext => {
console.log(oContext.getObject());
});
});
}
handleEdit: function(oEvent){
let oModel = this.getView().getModel();
let bookID = oEvent.getSource().getBindingContext().getObject().ID;
let oBindList = oModel.bindList("/Books");
let aFilter = new sap.ui.model.Filter("ID", sap.ui.model.FilterOperator.EQ, bookID);
oBindList.filter(aFilter).requestContexts().then(function (aContexts) {
aContexts[0].setProperty("description", "new description");
aContexts[0].setProperty("title", "Test");
});
},
handleDelete: function(oEvent){
let oModel = this.getView().getModel();
let bookID = oEvent.getParameters().listItem.getBindingContext().getObject().ID;
let oBindList = oModel.bindList("/Books");
let aFilter = new sap.ui.model.Filter("ID", sap.ui.model.FilterOperator.EQ, bookID);
oBindList.filter(aFilter).requestContexts().then(function (aContexts) {
aContexts[0].delete();
});
},
let oContextBinding = oModel.bindContext("/SomeEntity(ID='" + entityID + "')/set");
oContextBinding.requestObject().then(function (aContexts) {
console.log(aContexts);
}.bind(this), function (oError) {
console.log(oError);
});
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
21 | |
12 | |
9 | |
8 | |
8 | |
7 | |
7 | |
6 | |
6 | |
6 |