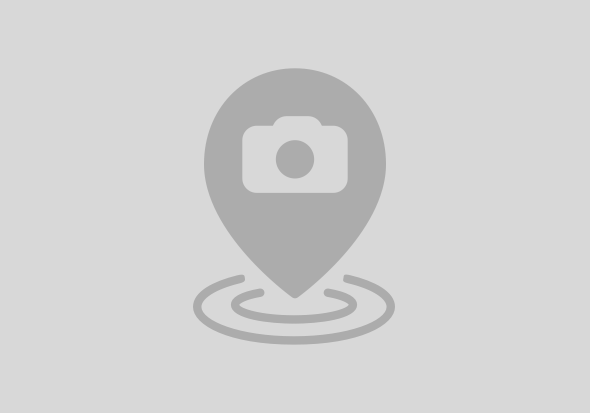
The Objective of this tutorial is to give you a walkthrough on how to create a web service for generating an Xml query, posting it to a server and getting the response. The created web service will be provided with a WSDL URL, which can be used in any application for consuming the web service.
The case here considered for creating the web service is tracking an item based on its GTIN and Serial Number or Barcode. Based on the GTIN and Serial Number a URN is formed and is given as input along with Query Type and Action Type to the web service, which returns the XML form of the query.
Let us consider ‘SimpleEventQuery’ as the Query Type and ‘MATCH_epc’ as the Action Type with the product having GTIN 10066800543215 and Serial Number 1308. The URN formed for the action ‘MATCH_epc’ will look like [urn:epc:id:sgtin:0066800.054321.1308]. And the XML form of query will be,
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:urn="urn:epcglobal:epcis-query:xsd:1">
<soapenv:Header/>
<soapenv:Body>
<urn: Poll>
<queryName>SimpleEventQuery</queryName>
<params>
<param>
<name>MATCH_epc</name>
<value>
<string>urn:epc:id:sgtin:0066800.054321.1308</string>
</value>
</param>
</params>
</urn: Poll>
</soapenv:Body>
</soapenv:Envelope>
Now, the generated XML query will be posted to server. To do this Server URL, UserName, Password along with the XML query are passed to web service as inputs and web service returns a string containing Response Number, Response and a Message separated by a delimiter “@@@”.
The libraries are required for using HTTP POST method.
Click here to know how to create EAR with External Library DC added to it.
The EAR application httpLibEAR with public part httplib is considered here for explanation purpose.
Select httpLibEAR under My Components and select Finish.
Repeat the same steps for querypostejb and add httpLibEAR to Dependencies.
If you are not able to see Component Properties in NWDS window,
Go to Windows → Show View → Other → select Component Properties and click OK
Then click OK.
This is the important step. If you miss this step you will get error during either build time or while deploying.
@Stateless(name = "QueryPost")
public class QueryPost implements QueryPostRemote, QueryPostLocal {
//@@begin javadoc:createXML()
/**
* Take the inputs and create the xml query
* @Param String query, String action, String urn
* @return String xml
*/
//@@end
public String createXML(String query, String action, String urn ) {
//@@begin createXML()
StringBuffer xml = new StringBuffer(); //to create the xml
/*=====generate XML=======*/
xml.append("<?xml version='1.0' encoding='UTF-8'?>\n")
.append("<soapenv:Envelope xmlns:soapenv='http://schemas.xmlsoap.org/soap/envelope/'\n")
.append("xmlns:urn='urn:epcglobal:epcis-query:xsd:1'>\n")
.append("<soapenv:Header/>\n")
.append("<soapenv:Body>\n")
.append("<urn:Poll>\n")
.append("<queryName>")
.append(query)
.append("</queryName>\n")
.append("<params>\n")
.append("<param>\n")
.append("<name>")
.append(action)
.append("</name>\n")
.append("<value>\n")
.append("<string>")
.append(urn)
.append("</string>\n")
.append("</value>\n")
.append("</param>\n")
.append("</params>\n")
.append("</urn:Poll>\n")
.append("</soapenv:Body>\n")
.append("</soapenv:Envelope>");
return xml.toString(); //return generated xml in string format
//@@end
}
//@@begin javadoc:postXML()
/**
* Take the XML query, Post it to the server and get response
* @Param String xml_query, String url, String userName, String password
* @return reply
*/
//@@end
@SuppressWarnings({ "finally", "deprecation" })
public String postXML( String xml_query, String url, String userName, String password ) {
//@@begin postXML()
String response = null; //to store the response from server
int result = 0;
String message = null;
String reply = null;
PostMethod post = new PostMethod(url); //initialize post method
post.setRequestHeader("Content-type", "text/xml; charset=UTF-8"); //set content type i.e. XML
post.setRequestBody(xml_query); //set query string to be posted
// Get HTTP client
HttpClient httpclient = new HttpClient();
httpclient.getParams().setAuthenticationPreemptive(true);
/*==authentication==*/
Credentials defaultcreds = new UsernamePasswordCredentials(userName, password);
try {
//post the XML execute and get response number
result = httpclient.executeMethod(post);
response = post.getResponseBodyAsString(); //get the response text
if(result==200){
message = "Success";
}else{
message = "Error: "+Integer.toString(result);
}
} catch (HttpException e) {
response = "null";
message = "Could not establish connection!";
} catch (IOException e) {
response = "null";
message = "Could not post your query, please try after sometime";
} finally {
// Release current connection to the connection pool once you are done
post.releaseConnection();
//Append result, response and message to the return string with delimiter "@@@".
reply = result+"@@@"+response+"@@@"+message;
return reply;
}
//@@end
}
}
There are two methods. CreateXML() and PostXML().
The XML Query is generated in the method CreateXML() & PostXML() method is used to post the generated XML Query to message server and get the response.
The Web Services wizard appears.
Choose Next.
The Service Endpoint Interface screen opens.
Choose Next.
Building and Deploying
Testing the Web Service
http://www.sdn.sap.com/irj/scn/go/portal/prtroot/docs/library/uuid/006a6229-b1ed-2e10-0c8c-cc5673cf2...
http://scn.sap.com/community/web-dynpro-java/blog/2013/09/02/javalangnoclassdeffounderror--not-again
http://www.sdn.sap.com/irj/scn/go/portal/prtroot/docs/library/uuid/b030e7fb-2662-2b10-0dab-c4aa52c35...
DC: Development Component
EAR: Enterprise ARchive
EJB: Enterprise Java Bean
EPC: Electronic Product Code
GTIN: Global Trade Identification Number
HTTP: Hyper Text Transfer Protocol
HTTPS: Hyper Text Transfer Protocol Secure
JAR: Java ARchive
NWDS: Net Weaver Development Studio
URL: Uniform Resource Locator
URN: Uniform Resource Name
WSDL: Web Services Description Language
XML: Extensible Markup Language
OER: Object Event Repository
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
10 | |
9 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |