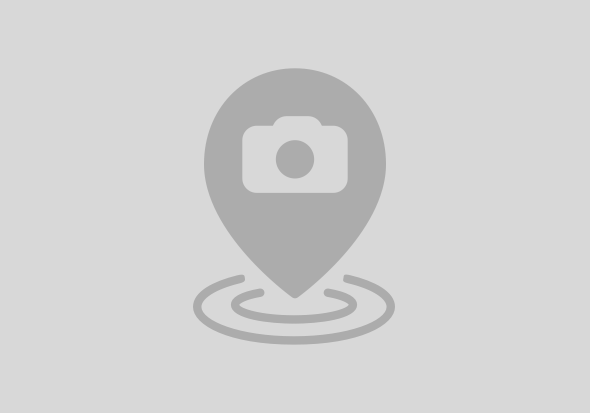
Equipment Hierarchy
Equipment | Parent_Equipment | Top_Level_Equipment |
10000028 | ||
10000029 | 10000028 | 10000028 |
10000030 | 10000029 | 10000028 |
10000031 | 10000029 | 10000028 |
10000032 | 10000029 | 10000028 |
@EndUserText.label: 'Equipment Hierarchy'
define table function zi_equi_hier_1
with parameters
@Environment.systemField: #CLIENT
mandt:abap.clnt
returns {
mandt : mandt;
equnr : equnr; // Equipment
pequi : equnr; // Parent Equipment
hequi : equnr; // Top Level Equipment
}
implemented by method zcl_get_hier=>get_top_hier;
class ZCL_GET_HIER definition
public
final
create public .
public section.
TYPES: BEGIN OF ty_equi,
equnr TYPE equnr,
hequi TYPE equnr,
END OF ty_equi,
BEGIN OF ty_equnr,
equnr TYPE equnr,
END OF ty_equnr,
tty_equi TYPE STANDARD TABLE OF ty_equi,
tty_equnr TYPE STANDARD TABLE OF ty_equnr.
data: lv_data type char1,
lv_equnr type equnr,
lv_hequi type equnr,
lwa_equi TYPE ty_equi,
lt_equi TYPE STANDARD TABLE OF ty_equi.
INTERFACES IF_AMDP_MARKER_HDB.
class-methods: GET_TOP_HIER FOR TABLE FUNCTION zi_equi_hier_1.
protected section.
private section.
class-methods: get_top EXPORTING VALUE(et_equi) TYPE tty_equi,
get_all_equnr EXPORTING VALUE(et_equnr) TYPE tty_equnr.
ENDCLASS.
METHOD get_all_equnr BY DATABASE PROCEDURE
FOR HDB LANGUAGE SQLSCRIPT
OPTIONS READ-ONLY USING equz.
declare lv_mandt "$ABAP.type( MANDT )" := session_context('CLIENT');
et_equnr = select equnr
from equz
where mandt = :lv_mandt
and hequi <> '';
endmethod.
METHOD get_top BY DATABASE PROCEDURE
FOR HDB LANGUAGE SQLSCRIPT
OPTIONS READ-ONLY USING equz ZCL_GET_HIER=>GET_ALL_EQUNR.
declare lv_mandt "$ABAP.type( MANDT )" := session_context('CLIENT');
DECLARE lv_dat int;
DECLARE lv_data int;
DECLARE lv_ind int;
DECLARE lv_equi char( 18 );
DECLARE lv_equnr char( 18 );
DECLARE lv_hequi char( 18 );
CALL "ZCL_GET_HIER=>GET_ALL_EQUNR"( et_equnr => lt_equi );
lv_ind = 1;
lv_data = 1;
while:lv_data = 1 DO
lv_dat = 1;
while:lv_dat = 1 DO
if :lv_equi = ''
then
lv_equi = :lt_equi.equnr[lv_ind];
lv_equnr = :lt_equi.equnr[lv_ind];
ELSEIF :lv_equi IS NULL
then
lv_equi = :lt_equi.equnr[lv_ind];
lv_equnr = :lt_equi.equnr[lv_ind];
END IF;
begin
lt_data = select hequi as hequi
from equz
where mandt = :lv_mandt and
equnr = :lv_equi;
lv_hequi = :lt_data.hequi[1];
if :lv_hequi = ''
then
et_equi.equnr[lv_ind] = :lv_equnr;
et_equi.hequi[lv_ind] = :lv_equi;
lv_dat = 0;
ELSE
lv_equi = lv_hequi;
END IF;
end;
end WHILE ;
lv_ind = lv_ind + 1;
lv_equi = :lt_equi.equnr[lv_ind];
if :lv_equi IS NULL
then
lv_data = 0;
else
lv_equi = '';
lv_equnr = '';
end if;
end WHILE ;
ENDMETHOD.
method GET_TOP_HIER BY DATABASE FUNCTION
FOR HDB LANGUAGE SQLSCRIPT
OPTIONS READ-ONLY USING equz ZCL_GET_HIER=>GET_TOP.
CALL "ZCL_GET_HIER=>GET_TOP"(et_equi => lt_equi);
RETURN
select eq.mandt as mandt,
eq.equnr as equnr,
eq.hequi as pequi,
teq.hequi as hequi
from equz as eq
LEFT OUTER JOIN :lt_equi as teq
ON teq.equnr = eq.equnr
where eq.mandt = :mandt;
endmethod.
@AbapCatalog.sqlViewName: 'ZEQUI_HIER2'
@AbapCatalog.compiler.compareFilter: true
@AbapCatalog.preserveKey: true
@AccessControl.authorizationCheck: #CHECK
@EndUserText.label: 'ZI_EQUI_HIER_1'
define view ZEQUI_HIER_2
as select from zi_equi_hier_1( mandt : $session.client ) as zeq
{
key zeq.equnr as Equipment,
zeq.pequi as Parent_Equipment,
zeq.hequi as Top_Level_Equipment
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
10 | |
9 | |
5 | |
4 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 |