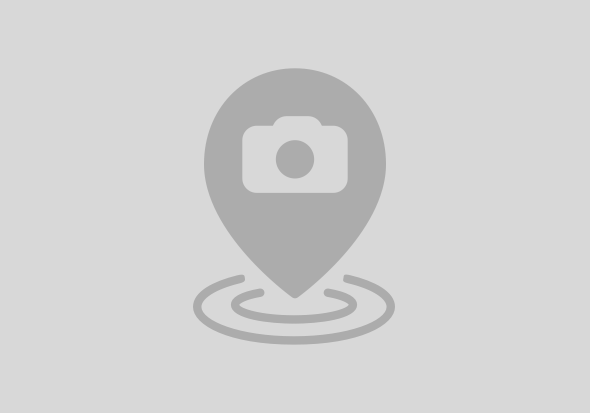
The HDI SQL APIs require the respective permissions to be granted to the invoking user. The provided example requires a database user with assigned HDI Container Group Administrator privileges for all needed Container Groups, e.g., for the default XSA-managed Container Group _SYS_DI#SYS_XS_HANA_BROKER.
Please review following steps provided as a reference to fulfill the requirements (please note that the list of granted permissions can be further narrowed down). The complete and comprehensive guidelines to manage SAP HANA HDI Containers are provided in SAP HANA Deployment Infrastructure (HDI) Reference for SAP HANA Platform.
CREATE USER HDI_ADMIN PASSWORD YOUR_PASSWORD NO FORCE_FIRST_PASSWORD_CHANGE;
CREATE USER CONTAINER_GROUP_ADMIN PASSWORD YOUR_PASSWORD NO FORCE_FIRST_PASSWORD_CHANGE;
CREATE LOCAL TEMPORARY TABLE #PRIVILEGES LIKE _SYS_DI.TT_API_PRIVILEGES;
INSERT INTO #PRIVILEGES (PRINCIPAL_NAME, PRIVILEGE_NAME, OBJECT_NAME) SELECT 'HDI_ADMIN', PRIVILEGE_NAME, OBJECT_NAME FROM _SYS_DI.T_DEFAULT_DI_ADMIN_PRIVILEGES;
CALL _SYS_DI.GRANT_CONTAINER_GROUP_API_PRIVILEGES('_SYS_DI', #PRIVILEGES, _SYS_DI.T_NO_PARAMETERS, ?, ?, ?);
DROP TABLE #PRIVILEGES;
CREATE LOCAL TEMPORARY COLUMN TABLE #PRIVILEGES LIKE _SYS_DI.TT_API_PRIVILEGES;
INSERT INTO #PRIVILEGES (PRINCIPAL_NAME, PRIVILEGE_NAME, OBJECT_NAME) SELECT 'CONTAINER_GROUP_ADMIN', PRIVILEGE_NAME, OBJECT_NAME FROM _SYS_DI.T_DEFAULT_CONTAINER_GROUP_ADMIN_PRIVILEGES;
CALL _SYS_DI.GRANT_CONTAINER_GROUP_API_PRIVILEGES('SYS_XS_HANA_BROKER', #PRIVILEGES, _SYS_DI.T_NO_PARAMETERS, ?, ?, ?);
DROP TABLE #PRIVILEGES;
CREATE LOCAL TEMPORARY COLUMN TABLE #DROP_CONTAINER_PARAMETERS LIKE _SYS_DI.TT_PARAMETERS;
INSERT INTO #DROP_CONTAINER_PARAMETERS ( KEY, VALUE ) VALUES ( 'ignore_work', 'true' );
INSERT INTO #DROP_CONTAINER_PARAMETERS ( KEY, VALUE ) VALUES ( 'ignore_deployed', 'true' );
CALL _SYS_DI#G.DROP_CONTAINER('C', #DROP_CONTAINER_PARAMETERS, ?, ?, ?);
DROP TABLE #DROP_CONTAINER_PARAMETERS;
hana-task-runner
├── components
│ ├── client.py
│ └── runner.py
├── config.yaml
├── requirements.txt
└── task_runner.py
connection:
indexserver_hostname: hostname # The indexserver hostname
indexserver_port: 30015 # The indexserver SQL port
container_group_admin: USER # The user with Container Group Administrator
password: PLAIN_PASSWORD # The password
encrypt: False # Keep False by default for not SSL-enforced connections
sslValidateCertificate: False # Keep False by default
client_config:
output_dir: ./output
logging_level: INFO # CRITICAL | ERROR | WARNING | INFO | DEBUG | NOTSET
processing:
max_concurrency: 3 # The allowed number of parallel operations
operations:
_SYS_DI#SYS_XS_HANA_BROKER: # The container group name
delete: # The operation to be performed, supported options: delete
- NAMED_CONTAINER # The container name
def get_database_connection(self):
try:
configuration = self.config['connection']
connection = dbapi.connect(
address = configuration['indexserver_hostname'],
port = configuration['indexserver_port'],
user = configuration['container_group_admin'],
password = configuration['password'],
encrypt = configuration['encrypt'],
sslValidateCertificate = configuration['sslValidateCertificate'],
communicationTimeout = 0)
except Exception as e: # pylint: disable=invalid-name
address = configuration['indexserver_hostname']
port = configuration['indexserver_port']
user = configuration['container_group_admin']
logging.error(
(f'Failed to establish connection to indexserver on {address}:{port} '
f'with user {user}'),
exc_info=e)
return None
else:
return connection
def drop_container(self, container_group_name, container_name):
try:
connection = self.get_database_connection()
cursor = connection.cursor()
except Exception as e: # pylint: disable=invalid-name
logging.error(
('Failed to establish connection to database when deleting '
f'container {container_name} from group {container_group_name}'),
exc_info=e)
else:
try:
logging.info((f'Started dropping container {container_name} '
f'from group {container_group_name}'))
cursor.execute(("create local temporary column table #drop_container_parameters "
"like _sys_di.tt_parameters;"))
cursor.execute(("insert into #drop_container_parameters ( key, value ) "
"values ( 'ignore_errors', 'true' );"))
cursor.execute(("insert into #drop_container_parameters ( key, value ) "
"values ( 'ignore_work', 'true' );"))
cursor.execute(("insert into #drop_container_parameters ( key, value ) "
"values ( 'ignore_deployed', 'true' );"))
cursor.execute((f"call {container_group_name}.drop_container('{container_name}', "
"#drop_container_parameters, ?, ?, ?);"))
keys = [ x[0] for x in cursor.description]
rows = cursor.fetchall()
result_set = pd.DataFrame(rows, columns=keys)
pretty_printed_results = tabulate(result_set, headers='keys', tablefmt='psql')
logging.info(
(
'Results of executing SQL: '
f'call {container_group_name}.drop_container(\'{container_name}\', '
f'#drop_container_parameters, ?, ?, ?))\n'
f'{pretty_printed_results}'
)
)
cursor.execute('drop table #drop_container_parameters;')
logging.info((f'Dropped container {container_name} '
f'from group {container_group_name}'))
except Exception as e: # pylint: disable=invalid-name
logging.error((
(f'Failed to drop container {container_name} '
f'from group {container_group_name}')
),exc_info=e)
finally:
cursor.close()
connection.close()
import asyncio
import logging
import time
class Runner:
def __init__(self, sem_value):
self.sem_value = sem_value
async def execute_task(self, semaphore, partial):
loop = asyncio.get_running_loop()
async with semaphore:
await loop.run_in_executor(None, partial)
async def execute_worklist(self, loop, partials):
semaphore = asyncio.Semaphore(value=self.sem_value)
worklist = []
for partial in partials:
worklist.append(self.execute_task(semaphore, partial))
logging.info(f'Limited to {self.sem_value} parallel tasks')
await asyncio.wait(worklist)
def run(self, partials):
start = time.time()
loop = asyncio.get_event_loop()
logging.info('Started parallel processing')
loop.run_until_complete(self.execute_worklist(loop, partials))
loop.close()
end = time.time()
logging.info(f'Finished parallel processing in {end-start}s')
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
6 | |
5 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 | |
3 | |
2 |