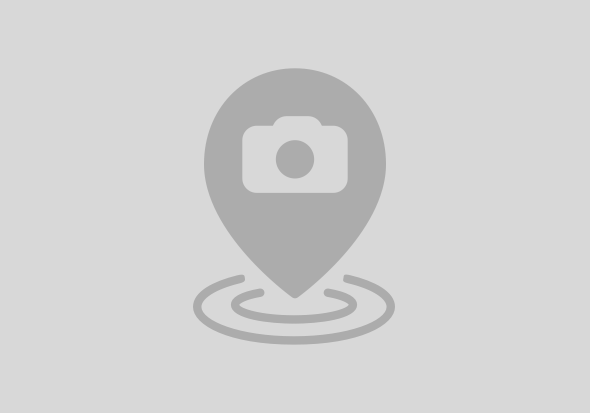
mkdir openaiapi
cd openaiapi
npm init
package name: (openaiapi) openaiapi
version: (1.0.0) 1.0.0
description: OpenAI based NodeJs API
entry point: (index.js) server.js
test command: node server
git repository:
keywords:
author: Kapil Verma [Put your name offcourse]
const express = require('express');
const bodyParser = require('body-parser');
const fs = require('fs');
const app = express();
var similarity = require( 'compute-cosine-similarity' );
const basicAuth = require('express-basic-auth');
var cors = require('cors');
const axios = require('axios');
const PORT = 3000;
const token = 'sk-XXXXXXXXXXXXXX|Your Secret KEy|XXXXXXXXXXXXXXXXXXXX';
Note: We are using Basic Auth here with Username: admin & Password: oursecret
// Data File
const dataFilePath = 'data.json';
// Middleware to parse incoming JSON data & CORS Handler
app.use(bodyParser.json());
app.use(cors())
//Adding Auth
app.use(basicAuth({
users: { 'admin': 'oursecret' }
}))
// Welcome Page
app.get('/', (req, res) => {
res.send('<p>Welcome to OPEN API Gateway</p><p>Service is Up & Running </p><p></p>' + Date());
})
// Start the server
app.listen(PORT, () => {
console.log(`OpenAI API is running on port ${PORT}`)
})
const express = require('express');
const bodyParser = require('body-parser');
const fs = require('fs');
const app = express();
var similarity = require( 'compute-cosine-similarity' );
const basicAuth = require('express-basic-auth');
var cors = require('cors');
const axios = require('axios');
const PORT = 3000;
const token = 'sk-XXXXXXXXXXXXXX|Your Secret KEy|XXXXXXXXXXXXXXXXXXXX';
// Data File
const dataFilePath = 'data.json';
// Middleware to parse incoming JSON data & Disable CORS
app.use(bodyParser.json());
app.use(cors())
//Adding Auth
app.use(basicAuth({
users: { 'admin': 'supersecret' }
}))
// Welcome Page
app.get('/', (req, res) => {
res.send('<p>Welcome to OPEN API Gateway</p><p>Service is Up & Running </p><p></p>' + Date());
})
// Start the server
app.listen(PORT, () => {
console.log(`OpenAI API is running on port ${PORT}`)
})
npm i express body-parser compute-cosine-similarity express-basic-auth cors axios
"start": "node server.js"
npm start
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
10 | |
9 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |