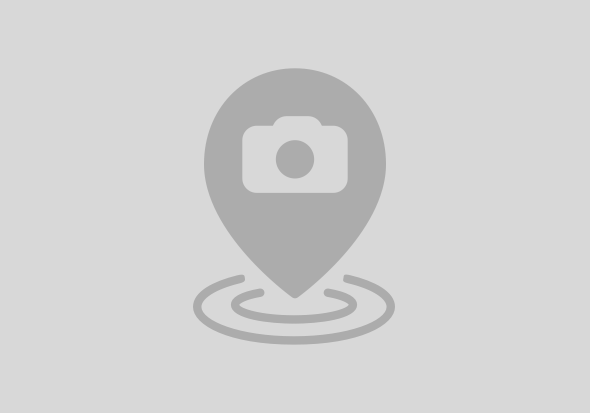
Important Note: The Purchasing (/IBP/API_PURCHASING) OData API service is currently only available by individual customer request. To use it, create an incident on component SCM-IBP-INT-ODT-PUR, requesting SAP to enable the communication scenario SAP_COM_0955.
Communication Arrangement
import requests
import pandas as pd
import json
import time
PlanningAreaID | VersionID | SourceLogicalSystem | IBPPurgDocIntegrationMode |
IBPPurgDocType | IBPPurgDocExt | IBPPurgDocItem | IBPPurgSOSAdditionalLaneID | IBPPurgSOSModeOfTransport | IBPGRProcgTmeInDays | IBPPurgDocScheduleLine |
ProductID | ShipToLocationID | ShipFromLocationID | IBPPurgDeliveryDateTime | IBPPurgOrderedQuantity | IBPPurgReceiptQuantity | IBPPurgDocQuantityUnit |
df1 = pd.read_excel(r"C:\Users\I573991\OneDrive - SAP SE\Python\ReadExternalFile\IBPPurgDocRootAsyncWrite.xlsx",sheet_name= 'Root')
df1.insert(0,"TransactionID",TransactionID)
df3['IBPPurgDeliveryDateTime'] = df3['IBPPurgDeliveryDateTime'].dt.strftime('%Y-%m-%dT%H:%M:%SZ')
df4 = (df3.groupby(["IBPPurgDocType","IBPPurgDocExt","IBPPurgDocItem","IBPPurgSOSAdditionalLaneID","IBPPurgSOSModeOfTransport","IBPGRProcgTmeInDays"],group_keys=True).apply(lambda x: x[["IBPPurgDocScheduleLine","ProductID","ShipToLocationID","ShipFromLocationID","IBPPurgDeliveryDateTime","IBPPurgOrderedQuantity","IBPPurgReceiptQuantity","IBPPurgDocQuantityUnit"]].to_dict('records'),include_groups = False).reset_index().rename(columns= {0:'_IBPPurgDocSchdLnAsyncWrite'}))
dfmerge = df1.merge(df4,'cross',None)
df5 = (dfmerge.groupby(['TransactionID','PlanningAreaID','VersionID','SourceLogicalSystem','IBPPurgDocIntegrationMode'],group_keys=True).apply(lambda x: x[["IBPPurgDocType","IBPPurgDocExt","IBPPurgDocItem","IBPPurgSOSAdditionalLaneID","IBPPurgSOSModeOfTransport","IBPGRProcgTmeInDays","_IBPPurgDocSchdLnAsyncWrite"]].to_dict('records'),include_groups = False).reset_index().rename(columns= {0:'_IBPPurgDocItemAsyncWrite'}))
j1 = (df5.to_json(orient='records',date_format = 'iso',indent =2,index=False))[1:-1]
{
"TransactionID":"33a06853-4c49-1eee-b2df-351a7e3b070f",
"PlanningAreaID":"SAP7FAC",
"VersionID":"__BASELINE",
"SourceLogicalSystem":"OBPSRC",
"IBPPurgDocIntegrationMode":"UPSERT",
"_IBPPurgDocItemAsyncWrite":[
{
"IBPPurgDocType":"PO_ITM",
"IBPPurgDocExt":"20001001",
"IBPPurgDocItem":"000010",
"IBPPurgSOSAdditionalLaneID":"0_0001_3_5300011243_000000",
"IBPPurgSOSModeOfTransport":"DEF",
"IBPGRProcgTmeInDays":2,
"_IBPPurgDocSchdLnAsyncWrite":[
{
"IBPPurgDocScheduleLine":"0010",
"ProductID":"GG03_BOARD_A@QKV002",
"ShipToLocationID":"PLFA71@QKV002",
"ShipFromLocationID":"SUSUPPLIER71@QKV002",
"IBPPurgDeliveryDateTime":"2024-03-26T00:00:00Z",
"IBPPurgOrderedQuantity":100,
"IBPPurgReceiptQuantity":100,
"IBPPurgDocQuantityUnit":"EA"
},
{
"IBPPurgDocScheduleLine":"0020",
"ProductID":"GG03_BOARD_A@QKV002",
"ShipToLocationID":"PLFA71@QKV002",
"ShipFromLocationID":"SUSUPPLIER71@QKV002",
"IBPPurgDeliveryDateTime":"2024-03-27T00:00:00Z",
"IBPPurgOrderedQuantity":200,
"IBPPurgReceiptQuantity":200,
"IBPPurgDocQuantityUnit":"EA"
}
]
},
{
"IBPPurgDocType":"PO_ITM",
"IBPPurgDocExt":"20001001",
"IBPPurgDocItem":"000020",
"IBPPurgSOSAdditionalLaneID":"0_0001_3_5300011243_000000",
"IBPPurgSOSModeOfTransport":"DEF",
"IBPGRProcgTmeInDays":2,
"_IBPPurgDocSchdLnAsyncWrite":[
{
"IBPPurgDocScheduleLine":"0010",
"ProductID":"GG03_BOARD_A@QKV002",
"ShipToLocationID":"PLFA71@QKV002",
"ShipFromLocationID":"SUSUPPLIER71@QKV002",
"IBPPurgDeliveryDateTime":"2024-02-28T00:00:00Z",
"IBPPurgOrderedQuantity":150,
"IBPPurgReceiptQuantity":150,
"IBPPurgDocQuantityUnit":"EA"
},
{
"IBPPurgDocScheduleLine":"0020",
"ProductID":"GG03_BOARD_A@QKV002",
"ShipToLocationID":"PLFA71@QKV002",
"ShipFromLocationID":"SUSUPPLIER71@QKV002",
"IBPPurgDeliveryDateTime":"2024-03-29T00:00:00Z",
"IBPPurgOrderedQuantity":250,
"IBPPurgReceiptQuantity":250,
"IBPPurgDocQuantityUnit":"EA"
}
]
},
{
"IBPPurgDocType":"PO_ITM",
"IBPPurgDocExt":"20002002",
"IBPPurgDocItem":"000010",
"IBPPurgSOSAdditionalLaneID":"0_0001_3_5300011243_000000",
"IBPPurgSOSModeOfTransport":"DEF",
"IBPGRProcgTmeInDays":2,
"_IBPPurgDocSchdLnAsyncWrite":[
{
"IBPPurgDocScheduleLine":"0010",
"ProductID":"GG03_BOARD_A@QKV002",
"ShipToLocationID":"PLFA71@QKV002",
"ShipFromLocationID":"SUSUPPLIER71@QKV002",
"IBPPurgDeliveryDateTime":"2024-03-30T00:00:00Z",
"IBPPurgOrderedQuantity":300,
"IBPPurgReceiptQuantity":300,
"IBPPurgDocQuantityUnit":"EA"
},
{
"IBPPurgDocScheduleLine":"0020",
"ProductID":"GG03_BOARD_A@QKV002",
"ShipToLocationID":"PLFA71@QKV002",
"ShipFromLocationID":"SUSUPPLIER71@QKV002",
"IBPPurgDeliveryDateTime":"2024-04-02T00:00:00Z",
"IBPPurgOrderedQuantity":350,
"IBPPurgReceiptQuantity":350,
"IBPPurgDocQuantityUnit":"EA"
}
]
}
]
}
3. Using Python Again, Making GET and POST requests to establish a connection with SAP IBP, get Transaction ID, and Post that JSON Payload to IBP calling the ODATA API service
#Define Your URL Here
SERVER_URL = ''
DATA_URL = f"https://{SERVER_URL}/sap/opu/odata4/ibp/api_purchasing/srvd_a2x/ibp/api_purchasing/0001/IBPPurgDocRootAsyncWrite"
#Provide UserName Password here
USERNAME = ''
PASSWORD = ''
data_get = requests.get(f"{DATA_URL}/SAP__self.GetTransactionID()",auth=(USERNAME, PASSWORD), verify=False,headers = {'x-csrf-token': 'Fetch'} )
if data_get.status_code == 200:
token = data_get.headers['x-csrf-token']
c = requests.utils.dict_from_cookiejar(data_get.cookies)
headers = {'x-csrf-token': token, 'Content-type': 'application/json'}
json1 = data_get.json()
df = pd.DataFrame.from_dict(pd.json_normalize(json1), orient='columns')
TransactionID = df['TransactionID'][0]
#POST JSON Payload
x = requests.post(DATA_URL,data=j4,auth=(USERNAME, PASSWORD), verify=False, headers = headers, cookies = c)
if x.status_code == 201:
#Commit the POST Request
y = requests.post(f"{DATA_URL}/SAP__self.Commit",data=j5,auth=(USERNAME, PASSWORD), verify=False, headers = headers, cookies = c )
if y.status_code == 200:
time.sleep(20)
status_get = requests.get(f"https://pt6-001-api.wdf.sap.corp/sap/opu/odata4/ibp/api_purchasing/srvd_a2x/ibp/api_purchasing/0001/IBPPurgDocRootAsyncWrite/SAP__self.GetStatus(TransactionID={TransactionID})",auth=(USERNAME, PASSWORD), verify=False, headers = headers, cookies = c)
print(status_get.text)
print(status_get.status_code)
{"@odata.context":"../$metadata#com.sap.gateway.srvd_a2x.ibp.api_purchasing.v0001.xIBPxD_PurgTransactionStatusR","@odata.metadataEtag":"W/\"20240213123138\"","TransactionStatus":"PROCESSED"}
200
status = requests.get("https://pt6-001-api.wdf.sap.corp/sap/opu/odata4/ibp/api_purchasing/srvd_a2x/ibp/api_purchasing/0001/IBPPurgDocMessageAsyncWrite",data=j5,auth=(USERNAME, PASSWORD), verify=False, headers = headers, cookies = c)
print(status.text)
Wrapping up, I would like to mention that I am not an expert in Python but I have tried to make use of the language to build a user-friendly utility here. There could be better ways of accomplishing the same task with better overall performance and one can do their code design.
I would appreciate it if you could leave your valuable thoughts and feedback for me to improve upon things here.
Regards
Gaurav Guglani
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
7 | |
6 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |