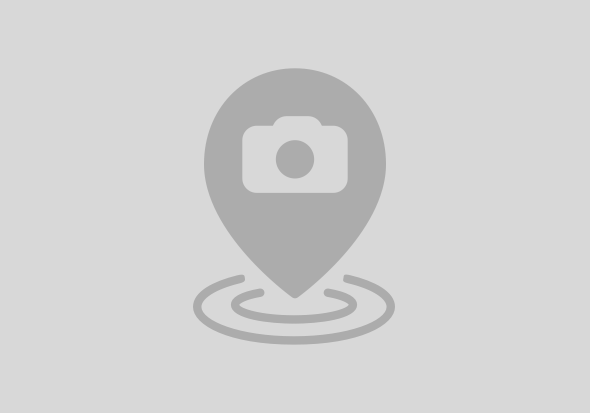
Consider the following fragment of coding.
DATA: v_matnr TYPE matnr,
v_ekgrp TYPE ekgrp,
v_count TYPE i,
v_value TYPE p LENGTH 8 DECIMALS 2.
...
PERFORM myform USING v_matnr v_ekgrp v_count v_value.
...
FORM myforum USING i_matnr
i_ekgrp
i_count
i_value.
DATA: l_result TYPE p LENGTH 12 DECIMALS 2.
l_result = i_count * i_value.
...
ENDFORM.
If I run the Extended Syntax Checker, I get the following message:
Program: ZMZPROG_STATUS Row: 57 Parameter "I_MATNR" is untyped.
Static type checks and optimizations,
therefore, cannot take place.
If a type cannot be declared, use the type ANY.
ANY. use the type ANY. use the type ANY.
use the type ANY.
Internal Message Code: MESSAGE GUX
(The message can be hidden with "#EC *)
Usually, it's a good idea, and probably part of your development procedures, to eliminate any such messages. So, what should we do for this one? One obvious action would be to make them all TYPE ANY. The message itself tells us that this will make the message go away. ( Using the comment "#EC * is definitely not the right way to proceed, and will probably get you into trouble with your quality team. Only suppress Extended Checker messages, when you can justify it from a programming point of view. )
You most definitely should not use TYPE ANY for typing your parameters. The reason why you should use specific types, is something called "type safety" - you ensure that the type of your calling parameter matches the called parameter at syntax check time. Errors picked up by the syntax checker are much more easily picked up than runtime errors.
Let's say I do use TYPE ANY, but make a mistake in my PERFORM statement, by getting some of the parameters the wrong way round. Like this:
DATA: v_matnr TYPE matnr,
v_ekgrp TYPE ekgrp,
v_count TYPE i,
v_value TYPE p LENGTH 8 DECIMALS 2.
...
PERFORM myform USING v_matnr v_count v_ekgrp v_value. " parms in wrong order
...
FORM myforum USING i_matnr TYPE ANY
i_ekgrp TYPE ANY
i_count TYPE ANY
i_value TYPE ANY.
DATA: l_result TYPE p LENGTH 12 DECIMALS 2.
l_result = i_count * i_value.
...
ENDFORM.
This will syntax check fine. But if you tried to run it, you'd probably get a dump about arithmetic conversion. Or worse, you wouldn't get a dump, but you'd get strange results - perhaps once the program is productive - that requires analysis and debugging to track down! If, on the other hand, I'd been more specific in my parameter types, like this:
DATA: v_matnr TYPE matnr,
v_ekgrp TYPE ekgrp,
v_count TYPE i,
v_value TYPE p LENGTH 8 DECIMALS 2.
...
PERFORM myform USING v_matnr v_count v_ekgrp v_value. " Parms in wrong order
...
FORM myforum USING i_matnr TYPE matnr
i_ekgrp TYPE ekgrp
i_count TYPE i
i_value TYPE numeric.
DATA: l_result TYPE p LENGTH 12 DECIMALS 2.
l_result = i_count * i_value.
...
ENDFORM.
Then a syntax check (just an ordinary one, not the Extended) would have picked up the error. An error picked up at syntax check time is much easier, and therefore cheaper, to fix than one picked up at runtime... when the program fails in production! An artificial example, sure. But if the FORM has a long list of parameters, you can see that it could easily happen! ( This is one reason why I much prefer programming in ABAP Objects - in that context, in most cases, the names of the parameters are visible in the CALLING code ).
So, when should you use TYPE ANY? Well, you'll notice in the definition of i_value, I could have TYPE p; or been even more specific and used a predefined type of p LENGTH 8 DECIMALS 2. But by defining them as NUMERIC the form can be used a v_value of any numeric type. That is, I, F or any form of P. This is design decision, according to the requirements of your program. Use type ANY when you really mean that the parameter could have any type. Also, have a look at the generic types - like NUMERIC, DATA, CLIKE
The general principle is to use as specific a type as possible in all your parameters. In this way, you'll have introduced type safety into your program, and your programs are less likely to have runtime errors.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
5 | |
5 | |
3 | |
3 | |
3 | |
2 | |
2 | |
2 | |
1 | |
1 |