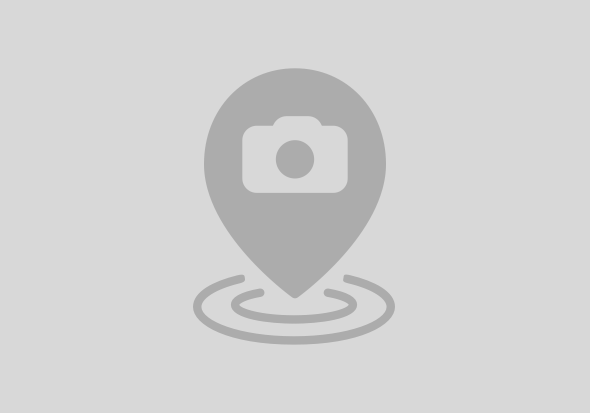
Note:
If you don't like reading, just scroll down to the end of this page to view the full source code.
Note:
If you don't like reading, just scroll down to the end of this page to view the full source code.
Map<String, Object> requestBody = request.getMapData();
Integer idToCreate = (Integer) requestBody.get("UniqueId");
String nameToCreate = (String) requestBody.get("Name");
createPerson(idToCreate, nameToCreate);
Note:
Of course, you know, when I say database, I mean any data store or any back end that you have in your individual enterprise scenario.
return CreateResponse.setSuccess().response();
Note:
For CREATE operation, the specification says that the created entity should be returned in the response of the POST request (as per default. We’ll discuss this in a future blog).
Furthermore, the status code is 201 (Created) upon successful completion
@Create(serviceName = "DemoService", entity = "People")
public CreateResponse createPerson(CreateRequest request) {
// extract the data for creation
Map<String, Object> requestBody = request.getMapData();
Integer idToCreate = (Integer) requestBody.get("UniqueId"); // we know it is an int, because we know the edmx
String nameToCreate = (String) requestBody.get("Name"); // same here
// do the actual creation in database
createPerson(idToCreate, nameToCreate);
return CreateResponse.setSuccess().response();
}
Note:
Of course, code is simplified, e.g. we're skipping the error handling, to make the code as simple as possible.
Ideally, we would e.g. check if the request body is valid, if the person already exists, etc
Integer idForUpdate = (Integer)request.getKeys().get("UniqueId");
Map<String, Object> requestBody = request.getMapData();
Map<String, Object> personMap = findPerson(idForUpdate);
Note:
Even if the user passes the key property in the request body: we cannot change it. The key field in the database cannot be modified, to ensure consistency/integrity of the database.
personMap.replace("Name", requestBody.get("Name"));
Note:
It would really really be better to check if the requested person exists at all...
You know how to do handle it, we've learned it in the previous blog, so we skip it here.
return UpdateResponse.setSuccess().response();
@Update(serviceName = "DemoService", entity = "People")
public UpdateResponse updatePerson(UpdateRequest request) {
// retrieve from request: which person to update
Integer idForUpdate = (Integer)request.getKeys().get("UniqueId");
// retrieve from request: the data to modify
Map<String, Object> requestBody = request.getMapData();
// retrieve from database: the person to modify
Map<String, Object> personMap = findPerson(idForUpdate);
// do the modification, but ignore key property (cannot be modified, to keep data consistent)
personMap.replace("Name", requestBody.get("Name"));
return UpdateResponse.setSuccess().response();
}
@Delete(serviceName = "DemoService", entity = "People")
public DeleteResponse deletePerson(DeleteRequest request) {
Integer id = (Integer)request.getKeys().get("UniqueId");
//find and remove the requested person
List<Map<String,Object>> peopleList = getPeople();
for (Iterator<Map<String, Object>> iterator = peopleList.iterator(); iterator.hasNext();) {
Map<String, Object> personMap = iterator.next();
if(((Integer)personMap.get("UniqueId")).equals(id)) {
iterator.remove();
break;
}
}
return DeleteResponse.setSuccess().response();
}
Note:
No notes this time…
{
"UniqueId": 4,
"Name": "Eric"
}
Note:
The value for UniqueId must be a number that doesn't exist in the People collection.
Remember, we don’t have a validation in our service implementation, neither we have an automatically generated UniqueId
name: Content-Type
value: application/json
Why?
Note:
The OData V4 library only supports json (currently) because the payload overhead is much smaller, so performance is better
Note:
If you're wondering, why do we get a response where the content is exactly what we've sent?
What is the benefit of this information?
Answer is, because in many cases, some values are generated by the back end, so the server has to inform the client about those values and also, how the new entry can be addressed (this info is in response header)
<?xml version="1.0" encoding="utf-8"?>
<edmx:Edmx Version="4.0" xmlns:edmx="http://docs.oasis-open.org/odata/ns/edmx">
<edmx:DataServices>
<Schema Namespace="demo" xmlns="http://docs.oasis-open.org/odata/ns/edm">
<EntityType Name="Person">
<Key>
<PropertyRef Name="UniqueId" />
</Key>
<Property Name="UniqueId" Type="Edm.Int32" />
<Property Name="Name" Type="Edm.String" />
</EntityType>
<EntityContainer Name="container" >
<EntitySet Name="People" EntityType="demo.Person" />
</EntityContainer>
</Schema>
</edmx:DataServices>
</edmx:Edmx>
package com.example.DemoService;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.sap.cloud.sdk.service.prov.api.operations.Create;
import com.sap.cloud.sdk.service.prov.api.operations.Delete;
import com.sap.cloud.sdk.service.prov.api.operations.Query;
import com.sap.cloud.sdk.service.prov.api.operations.Read;
import com.sap.cloud.sdk.service.prov.api.operations.Update;
import com.sap.cloud.sdk.service.prov.api.request.CreateRequest;
import com.sap.cloud.sdk.service.prov.api.request.DeleteRequest;
import com.sap.cloud.sdk.service.prov.api.request.QueryRequest;
import com.sap.cloud.sdk.service.prov.api.request.ReadRequest;
import com.sap.cloud.sdk.service.prov.api.request.UpdateRequest;
import com.sap.cloud.sdk.service.prov.api.response.CreateResponse;
import com.sap.cloud.sdk.service.prov.api.response.DeleteResponse;
import com.sap.cloud.sdk.service.prov.api.response.ErrorResponse;
import com.sap.cloud.sdk.service.prov.api.response.QueryResponse;
import com.sap.cloud.sdk.service.prov.api.response.ReadResponse;
import com.sap.cloud.sdk.service.prov.api.response.UpdateResponse;
public class ServiceImplementation {
private static List<Map<String, Object>> peopleList = null;
@Query(serviceName="DemoService", entity="People")
public QueryResponse getPeople(QueryRequest request) {
List<Map<String, Object>> peopleMap = getPeople();
return QueryResponse.setSuccess().setDataAsMap(peopleMap).response();
}
@Read(serviceName="DemoService", entity="People")
public ReadResponse getPerson(ReadRequest request) {
Integer id = (Integer)request.getKeys().get("UniqueId");
Map<String, Object> personMap = findPerson(id);
return ReadResponse.setSuccess().setData(personMap).response();
}
@Create(serviceName = "DemoService", entity = "People")
public CreateResponse createPerson(CreateRequest request) {
// extract the data for creation
Map<String, Object> requestBody = request.getMapData();
Integer idToCreate = (Integer) requestBody.get("UniqueId"); // we know it is an int, because we know the edmx
String nameToCreate = (String) requestBody.get("Name"); // same here
// do the actual creation in database
createPerson(idToCreate, nameToCreate);
return CreateResponse.setSuccess().response();
}
@Update(serviceName = "DemoService", entity = "People")
public UpdateResponse updatePerson(UpdateRequest request) {
// retrieve from request: which person to update
Integer idForUpdate = (Integer)request.getKeys().get("UniqueId");
// retrieve from request: the data to modify
Map<String, Object> requestBody = request.getMapData();
// retrieve from database: the person to modify
Map<String, Object> personMap = findPerson(idForUpdate);
// do the modification, but ignore key property (cannot be modified, to keep data consistent)
personMap.replace("Name", requestBody.get("Name"));
return UpdateResponse.setSuccess().response();
}
@Delete(serviceName = "DemoService", entity = "People")
public DeleteResponse deletePerson(DeleteRequest request) {
Integer id = (Integer)request.getKeys().get("UniqueId");
//find and remove the requested person
List<Map<String,Object>> peopleList = getPeople();
for (Iterator<Map<String, Object>> iterator = peopleList.iterator(); iterator.hasNext();) {
Map<String, Object> personMap = iterator.next();
if(((Integer)personMap.get("UniqueId")).equals(id)) {
iterator.remove();
break;
}
}
return DeleteResponse.setSuccess().response();
}
/* Dummy Data Store */
private List<Map<String, Object>> getPeople(){
// init the "database"
if(peopleList == null) {
peopleList = new ArrayList<Map<String, Object>>();
createPerson(0, "Anna");
createPerson(1, "Berta");
createPerson(2, "Claudia");
createPerson(3, "Debbie");
}
return peopleList;
}
private void createPerson(Integer id, String name){
Map<String, Object> personMap = new HashMap<String, Object>();
personMap.put("UniqueId", id);
personMap.put("Name", name);
peopleList.add(personMap);
}
private Map<String, Object> findPerson(Integer requiredPersonId){
List<Map<String,Object>> peopleList = getPeople();
for(Map<String, Object> personMap : peopleList) {
if(((Integer)personMap.get("UniqueId")).equals(requiredPersonId)) {
return personMap;
}
}
return null;
}
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
43 | |
25 | |
17 | |
15 | |
11 | |
7 | |
7 | |
6 | |
6 | |
6 |