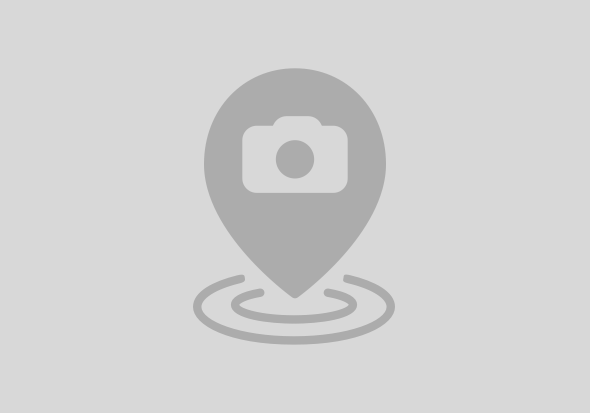
import pandas as pd
data_file = "Census01.csv"
data_folder = r"C:\Program Files\SAP Predictive Analytics\Desktop\Automated\Samples\Census"
df = pd.read_csv(data_folder + "\\" + data_file, header=0)
text= "Size of %s" % (data_file)
print('\x1b[1m'+ text + '\x1b[0m')
num = df.shape[0]
print("{} Rows ".format(num))
num = df.shape[1]
print("{} Columns ".format(num))
df.head(10)
s1=df['class'].value_counts()
s2=df['class'].value_counts(normalize = True) *100
dfc = pd.concat([s1.rename("Observations"), s2.rename("In %")], axis=1)
dfc['In %'] = dfc['In %'].round(2)
dfc.index.name = 'Class'
dfc
pd.crosstab(df['relationship'],df['class'],margins=True, normalize=True).round(4)*100
print('\x1b[1m'+ 'Python Version' + '\x1b[0m')
import platform
platform.python_version()
import sys
sys.path.append(r"C:\Program Files\SAP Predictive Analytics\Desktop\Automated\EXE\Clients\Python35")
import os
os.environ['PATH'] = r"C:\Program Files\SAP Predictive Analytics\Desktop\Automated\EXE\Clients\CPP"
AA_DIRECTORY = "C:\Program Files\SAP Predictive Analytics\Desktop\Automated"
import aalib
class DefaultContext(aalib.IKxenContext):
def __init__(self):
super().__init__()
def userMessage(self, iSource, iMessage, iLevel):
print(iMessage)
return True
def userConfirm(self, iSource, iPrompt):
pass
def userAskOne(iSource, iPrompt, iHidden):
pass
def stopCallBack(iSource):
pass
frontend = aalib.KxFrontEnd([])
factory = frontend.getFactory()
context = DefaultContext()
factory.setConfiguration("DefaultMessages", "true")
config_store = factory.createStore("Kxen.FileStore")
config_store.setContext(context, 'en', 10, False)
config_store.openStore(AA_DIRECTORY + "\EXE\Clients\CPP", "", "")
config_store.loadAdditionnalConfig("KxShell.cfg")
model = factory.createModel("Kxen.SimpleModel")
model.setContext(context, 'en', 8, False)
model.pushTransformInProtocol("Default", "Kxen.RobustRegression")
store = model.openNewStore("Kxen.FileStore", data_folder, "", "")
model.newDataSet("Training", data_file, store)
# model.guessSpaceDescription("Training")
metadata_file = "Desc_Census01.csv"
model.readSpaceDescription("Training", metadata_file, store)
target_col = list(df)[-1]
model.getParameter("")
variables = model.getParameter("Protocols/Default/Variables")
variables.setAllValues("Role", "input")
variables.setSubValue(target_col + "/Role", "target")
variables.setSubValue("KxIndex/Role", "skip")
model.validateParameter()
model.getParameter("")
model.changeParameter("Parameters/CutTrainingPolicy", "random with no test")
model.validateParameter()
model.getParameter("")
model.changeParameter("Protocols/Default/Transforms/Kxen.RobustRegression/Parameters/VariableSelection", "true")
model.validateParameter()
model.getParameter("")
model.changeParameter("Protocols/Default/Transforms/Kxen.RobustRegression/Parameters/Order", "1")
model.validateParameter()
model.sendMode(aalib.Kxen_learn, store)
help(model.saveModel)
model_folder = r"O:\MODULES_PA/PYTHON_API/MY_MODELS"
model_file = "models_space"
model.setName("My Classification Model")
model_comment = "Generated with Python API from Jupyter Notebook"
model_store = model.openNewStore("Kxen.FileStore", model_folder, "", "")
model.saveModel(model_store, model_file, model_comment)
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
18 | |
15 | |
11 | |
9 | |
9 | |
8 | |
8 | |
8 | |
7 | |
7 |