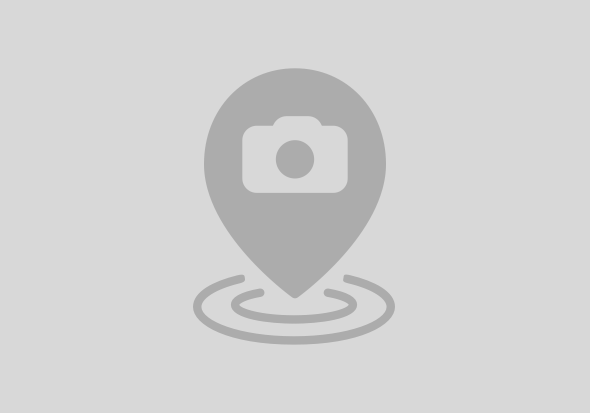
// Init canvas
function init() {
// Get the canvas and obtain a context for
// drawing in it
canvas = document.getElementById("myCanvas");
ctx = canvas.getContext('2d');
}
// Take image from webcam -> Data is stored in a variable window.filebinary
function snapshot() {
let image = new Image();
ctx.drawImage(video, 0,0, canvas.width, canvas.height);
var data = canvas.toDataURL('image/jpeg');
image.src = data;
window.filebinary = data;
}
sap.ui.define(["sap/ui/core/mvc/Controller"], function(BaseController) {
"use strict";
return BaseController.extend("ml001.app.controller.webcam", {
_onButtonPress1: function() {
this.getView().setBusy(true);
var token1 = "";
var out;
var xhr = new window.XMLHttpRequest();
var ifr = this.getView().byId("attachmentframe");
var file_bin = ifr.getDomRef().contentWindow.filebinary;
// GET X-CSRF-TOKEN - Hasan Rafiq
$.ajax({
url: '/sap/opu/odata/sap/<ZSERVICE>/',
type: 'GET',
async: false,
contentType: "application / atom + xml;type\ x3dentry;",
dataType: "",
headers: {
"x-csrf-token": "fetch"
},
success: function(data, textStatus, request) {
token1 = request.getResponseHeader("x-csrf-token");
}
});
// Post file binary to GW attachment service - Custom Hasan Rafiq
$.ajax({
type: 'POST',
url: '/sap/opu/odata/sap/<ZGW_SERVICE>/getimageSet',
async: false,
xhr: function() {
//Upload progress
xhr.upload.addEventListener('progress', function(evt) {
if (evt.lengthComputable) {
var percentComplete = Math.round(evt.loaded * 100 / evt.total);
$('.progress').val(percentComplete);
//Do something with upload progress
console.log(percentComplete);
}
}, false);
xhr.addEventListener('error', function(evt) {
this.getView().setBusy(true);
alert('There was an error attempting to upload the file.');
return;
}, false);
return xhr;
},
contentType: 'image/jpeg',
processData: false,
data: file_bin,
headers: {
'x-csrf-token': token1
},
success: function(data) {
//this.getView().setBusy(true);
//alert('File uploaded successfully');
out = data.documentElement.innerHTML;
}
});
//
if ( out )
{
sap.ui.controller("ml001.app.controller.ee6f9677cf93c15a0d5cde1b5_S5").manualUploadComplete(out);
sap.ui.controller("ml001.app.controller.ProductResult").fillValues();
var dialogName = "ProductResult";
var dialog = window.dialogs[dialogName];
dialog.open();
}
else
{
alert("Error in calling ML service");
}
var oDialog = this.getView().getContent()[0];
oDialog.close();
}
});
}, /* bExport= */
true);
#Hasan -> Run existing Transferlearning Tflow PB to classify new images
import numpy as np
import tensorflow as tf
import argparse
imagePath = 'image_to_classify/test.jpg'
modelFullPath = 'output_graph.pb'
labelsFullPath = 'output_labels.txt'
# Function to load existing tensor flow graph
def load_existing_tf_graph():
with tf.gfile.FastGFile(modelFullPath, 'rb') as f:
graph_def = tf.GraphDef()
graph_def.ParseFromString(f.read())
_ = tf.import_graph_def(graph_def, name='')
# Run existing graph on new image
def classify_image():
#Read image file into binary
image_data = tf.gfile.FastGFile(imagePath, 'rb').read()
#Run TF Session -> classifier
with tf.Session() as sess:
softmax_tensor = sess.graph.get_tensor_by_name('final_result:0')
predictions = sess.run(softmax_tensor,
{'DecodeJpeg/contents:0': image_data})
predictions = np.squeeze(predictions)
#Convert prediction probability vector to label texts ( Trained on )
f = open(labelsFullPath, 'rb')
lines = f.readlines()
labels = [str(w).replace("\n", "") for w in lines]
for x in range(0,predictions.shape[0]):
human_string = labels[x]
score = predictions[x]
print('%s (score = %.5f)' % (human_string, score))
# Main thread
if __name__ == '__main__':
load_existing_tf_graph()
classify_image()
{
"Hasan": 0.99126,
"Not_hasan": 0.00874
}
DATA: lo_http_client TYPE REF TO if_http_client,
lo_rest_client TYPE REF TO cl_rest_http_client,
lv_url TYPE string,
http_status TYPE string,
lv_body TYPE string.
DATA lr_json_deserializer TYPE REF TO cl_trex_json_deserializer.
TYPES: BEGIN OF ty_json_res,
hasan type string,
not_hasan type string,
END OF ty_json_res.
DATA: json_res TYPE ty_json_res.
cl_http_client=>create_by_destination(
EXPORTING
destination = 'MLRFC' " Logical destination (specified in function call)
IMPORTING
client = lo_http_client " HTTP Client Abstraction
EXCEPTIONS
argument_not_found = 1
destination_not_found = 2
destination_no_authority = 3
plugin_not_active = 4
internal_error = 5
OTHERS = 6
).
CREATE OBJECT lo_rest_client
EXPORTING
io_http_client = lo_http_client.
lo_http_client->request->set_version( if_http_request=>co_protocol_version_1_0 ).
IF lo_http_client IS BOUND AND lo_rest_client IS BOUND.
lv_url = 'infer'.
cl_http_utility=>set_request_uri(
EXPORTING
request = lo_http_client->request " HTTP Framework (iHTTP) HTTP Request
uri = lv_url " URI String (in the Form of /path?query-string)
).
lo_request = lo_rest_client->if_rest_client~create_request_entity( ).
lo_request->set_string_data( lv_body ).
lo_rest_client->if_rest_resource~post( lo_request ).
**-- Response
lo_response = lo_rest_client->if_rest_client~get_response_entity( ).
response = lo_response->get_string_data( ).
**-- Parse JSON
CREATE OBJECT lr_json_deserializer.
lr_json_deserializer->deserialize( EXPORTING json = response IMPORTING abap = json_res ).
**-- Here your structure JSON_RES should match output type
copy_data_to_ref( exporting is_data = json_res
changing cr_data = er_entity )
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
7 | |
5 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |