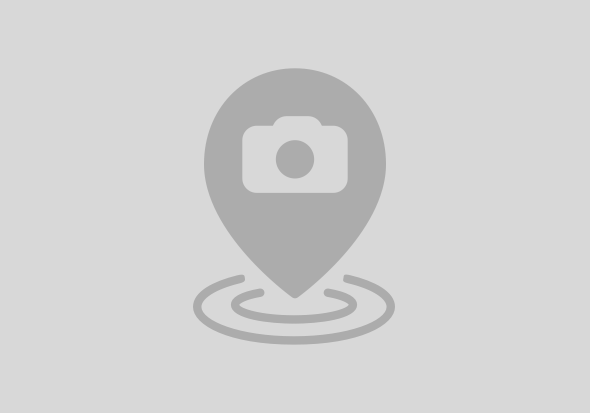
This is the 3rd and final part of this blog series. In my previous two blog posts I tried to depict the functionalities of new Tomcat 8 runtime. Also in the 2nd part of this series I have explained the non blocking IO functionality which comes as part of Servlet 3.1 in Tomcat 8 runtime. You can refer to the previous posts here -
Tomcat 8 runtime in HCP - Part 2 - Servlet 3.1
Now in this post I will explain another powerful feature that comes in this runtime i.e. HTTP protocol upgrade. With this functionality you can upgrade the protocol at runtime. The mechanism works much in the same way as web socket where the communication starts on HTTP and then using the same transport layer the communication protocol gets upgraded to Web Socket (ws) or Web Socket Secure (wss in case of SSL). However this upgrade functionality gives you more felxibility as you can implement your own protocol and implement it.
Let's jump in the code and have a look how the code works:
My project structure looks like below:
For this we created a Servlet and within this I am reading the header property "Upgrade". If the Upgrade request is for protocol "XXXX" I am requesting for upgrading the protocol and sending a HTTP 101 to the original HTTP response.
My Servlet code looks like below:
@WebServlet("/XXXXUpgradeServlet")
public class XXXXUpgradeServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
public void doGet(HttpServletRequest request,
HttpServletResponse response) {
if ("XXXX".equals(request.getHeader("Upgrade"))) {
/* Accept upgrade request */
response.setStatus(101);
response.setHeader("Upgrade", "XXXX");
response.setHeader("Connection", "Upgrade");
response.setHeader("OtherHeaderB", "Value");
/* Delegate the connection to the upgrade handler */
try {
XXXXUpgradeHandler XXXXUpgradeHandler = request.upgrade(XXXXUpgradeHandler.class);
} catch (IOException | ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
/* (the service method returns immedately) */
} else {
/* ... write error response ... */
}
}
}
So once the upgrade handler requests for upgrade you need to call the method "upgrade" and pass the implementation class that implements interface:
javax.servlet.http.HttpUpgradeHandler;
Here is the code for you:
public class XXXXUpgradeHandler implements HttpUpgradeHandler {
@Override
public void destroy() {
// TODO Auto-generated method stub
}
@Override
public void init(WebConnection wc) {
try {
ServletInputStream input = wc.getInputStream();
ServletOutputStream output = wc.getOutputStream();
/* ... implement XYZP using these streams (protocol-specific) ... */
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
In this code snippet there is no implementation of the new protocol. However this gives you the framework within which you can implement your own protocol.
Testing:
In order to test this please use Advanced rest control and run the servlet. You need to pass on Header parameter Upgrade=XXXX and you should be able to get a HTTP 101 response back.
In my case the parameters that need to pass in the HTTP call is as follows:
URL: http://localhost:8080/tc8/XXXXUpgradeServlet
HTTP Header Parameter:
Upgrade = XXXX
Connection = Upgrade
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
35 | |
21 | |
17 | |
15 | |
11 | |
9 | |
8 | |
8 | |
8 | |
7 |