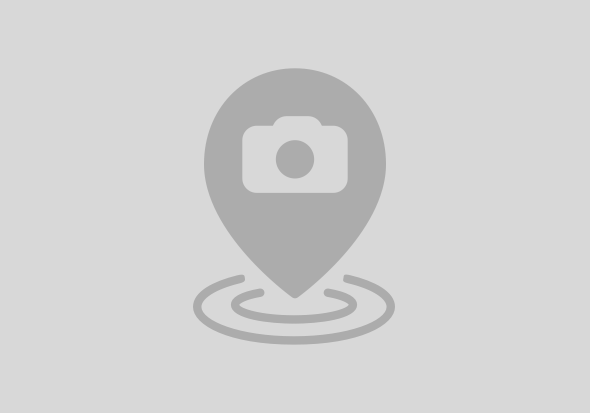
Public Sub changeUniverseAll()
Dim boDocId, boUnivSrcId, boUnivTgtId, rownum As Integer
Call logon
boUnivSrcId = Sheets("config").Cells(6, 2).value
boUnivTgtId = Sheets("config").Cells(7, 2).value
rownum = 2
While Sheets("liste docs").Cells(rownum, 1) <> ""
If Sheets("liste docs").Cells(rownum, 5) = "x" Then
boDocId = Sheets("liste docs").Cells(rownum, 1)
Call changeUniverseDoc(boDocId, boUnivSrcId, boUnivTgtId)
End If
rownum = rownum + 1
Wend
Call logoff
End Sub
<dataproviders>
<dataprovider>
<id>DP0</id>
<name>Query 1</name>
<dataSourceId>6187</dataSourceId>
<dataSourceType>unv</dataSourceType>
<updated>2006-09-20Z</updated>
</dataprovider>
<dataprovider>
<id>DP1</id>
<name>Query 2</name>
<dataSourceId>6191</dataSourceId>
<dataSourceType>unx</dataSourceType>
<updated>2014-04-23T09:08:20.000+02:00</updated>
</dataprovider>
<dataprovider>
<id>DP2</id>
<name>Query 3</name>
<updated>2014-04-23T09:08:20.000+02:00</updated>
</dataprovider>
</dataproviders>
Private Sub changeUniverseDoc(ByVal idDoc As Integer, ByVal idUnvSrc As Integer, ByVal idUnvTgt As Integer)
Dim objHTTP As WinHttp.WinHttpRequest
Dim objXML As MSXML2.DOMDocument
Dim oNodeXML, oSubNodeXML As MSXML2.IXMLDOMNode
Dim n As Integer
Dim dpType, dpId, dpSrcId, idUnv As String
Set objHTTP = CreateObject("WinHttp.WinHttpRequest.5.1")
Set objXML = CreateObject("Microsoft.XMLDOM")
url = boUrlRL & "/documents/" & idDoc & "/dataproviders"
objHTTP.Open "GET", url, False
objHTTP.SetRequestHeader "Content-type", "application/xml"
objHTTP.SetRequestHeader "Accept", "application/xml"
objHTTP.SetRequestHeader "X-SAP-LogonToken", boToken
objHTTP.Send ""
If objHTTP.Status <> "200" Then
Call afficheErrorREST(objHTTP, "getNumberDPLinkUnv", "Error gettin number of DP for document " & idDoc)
Call logoff
End
End If
objXML.LoadXML (objHTTP.ResponseText)
Debug.Print objHTTP.ResponseText
n = 0
On Error Resume Next ' car des fois le XML est mal formulé ...
For Each oNodeXML In objXML.SelectNodes("/dataproviders/dataprovider")
dpType = oNodeXML.SelectSingleNode("dataSourceType").Text
dpSrcId = oNodeXML.SelectSingleNode("dataSourceId").Text
dpId = oNodeXML.SelectSingleNode("id").Text
Debug.Print "Check DP " & dpId
If dpSrcId = idUnvSrc And dpType = "unv" Then
n = n + 1
Debug.Print "Change DP " & dpId
Call changeUniverseDocDP(idDoc, idUnvSrc, idUnvTgt, dpId)
'If dpId = "" Then Debug.Print "pas de dataSourceId pour le doc " & idDoc & " (" & dpType & ")"
End If
Next
On Error GoTo 0
If n = 0 Then
Call MsgBox("No change for doc " & idDoc, vbCritical)
Else
Call MsgBox("Doc " & idDoc & " changed", vbOKOnly)
End If
Set objXML = Nothing
Set objHTTP = Nothing
End Sub
<?xml version="1.0" encoding="UTF-8" standalone="yes" ?>
<mappings>
<content>
<mapping status="Ok">
<source>
<id>DS0.DOa6</id>
</source>
<target>
<id>DS1.DOa6</id>
</target>
</mapping>
<mapping status="Ok">
<source>
<id>DS0.DOda</id>
</source>
<target>
<id>DS1.DOda</id>
</target>
</mapping>
</content>
</mappings>
<?xml version="1.0" encoding="UTF-8" standalone="yes" ?>
<success>
<message>The resource of type 'Document' with identifier '12345' has been successfully updated.</message>
<id>10113</id>
</success>
Private Sub changeUniverseDocDP(idDoc As Integer, idUnvSrc As Integer, idUnvTgt As Integer, ByVal idDP As String)
Dim objHTTP As WinHttp.WinHttpRequest
Dim objXML As MSXML2.DOMDocument
Dim oNodeXML, oSubNodeXML As MSXML2.IXMLDOMNode
Dim n As Integer
Dim mappingstatus, idSource As String
Set objHTTP = CreateObject("WinHttp.WinHttpRequest.5.1")
Set objXML = CreateObject("Microsoft.XMLDOM")
' get & verify status of default mapping
url = boUrlRL & "/documents/" & idDoc & "/dataproviders/mappings?originDataproviderIds=" & idDP & "&targetDatasourceId=" & idUnvTgt
objHTTP.Open "GET", url, False
objHTTP.SetRequestHeader "Content-type", "application/xml"
objHTTP.SetRequestHeader "Accept", "application/xml"
objHTTP.SetRequestHeader "X-SAP-LogonToken", boToken
objHTTP.Send ""
If objHTTP.Status <> "200" Then
Call afficheErrorREST(objHTTP, "changeUniverseDocDP", "Error gettin default mapping for document " & idDoc & " & DP " & idDP)
Call logoff
End
End If
'verify default mapping
objXML.LoadXML (objHTTP.ResponseText)
n = 0
For Each oNodeXML In objXML.SelectNodes("/mappings/content/mapping")
mappingstatus = oNodeXML.Attributes.getNamedItem("status").Text
If mappingstatus <> "Ok" Then
n = n + 1
idSource = oNodeXML.SelectSingleNode("source/id").Text
Call MsgBox("Can't change mapping for Doc " & idDoc & ", DP " & idDP & ", column " & idSource & ", status=" & mappingstatus, vbCritical)
End If
Next
If n > 0 Then
Call MsgBox("Stop, can't change mapping")
Call logoff
End
End If
'commit
url = boUrlRL & "/documents/" & idDoc & "/dataproviders/mappings?originDataproviderIds=" & idDP & "&targetDatasourceId=" & idUnvTgt
objHTTP.Open "POST", url, False
objHTTP.SetRequestHeader "Content-type", "application/xml"
objHTTP.SetRequestHeader "Accept", "application/xml"
objHTTP.SetRequestHeader "X-SAP-LogonToken", boToken
objHTTP.Send objXML.XML
If objHTTP.Status <> "200" Then
Call afficheErrorREST(objHTTP, "changeUniverseDocDP", "Error commit mapping for document " & idDoc & " & DP " & idDP)
Call logoff
End
End If
objXML.LoadXML (objHTTP.ResponseText)
n = 0
For Each oNodeXML In objXML.SelectNodes("/success")
n = n + 1
Next
If n = 0 Then
Call MsgBox("Error on change mapping, no success ?")
End If
'save
url = boUrlRL & "/documents/" & idDoc
objHTTP.Open "PUT", url, False
objHTTP.SetRequestHeader "Content-type", "application/xml"
objHTTP.SetRequestHeader "Accept", "application/xml"
objHTTP.SetRequestHeader "X-SAP-LogonToken", boToken
objHTTP.Send ""
If objHTTP.Status <> "200" Then
Call afficheErrorREST(objHTTP, "changeUniverseDocDP", "Error saving document " & idDoc)
Call logoff
End
End If
Set objXML = Nothing
Set objHTTP = Nothing
End Sub
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
6 | |
5 | |
5 | |
5 | |
4 | |
4 | |
4 | |
4 | |
4 | |
3 |