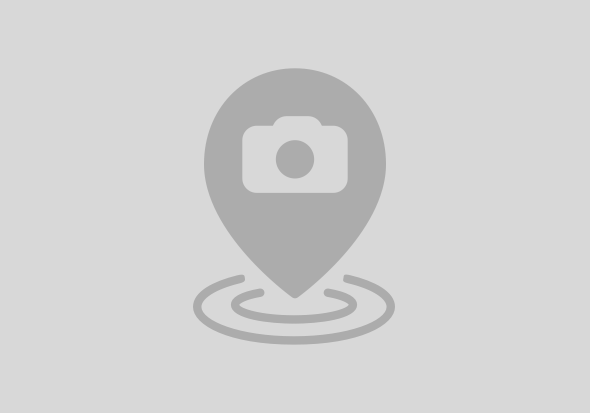
keys within translated text
{i18n>cleverfox}
in an XMLView or when using bundle.getText("cleverfox")
from JavaScript, we expect to have the following result:The quick brown fox jumps over the lazy dog.
sap.ui.define([
"sap/ui/model/resource/ResourceModel"
], function(ResourceModel) {
"use strict";
var DeepResourceModel = ResourceModel.extend("com.sample.DeepResourceModel", {
getProperty: function( sPath ) {
// call the original implementation
let s = ResourceModel.prototype.getProperty.call( this, sPath );
// do a little modification
return s.toUpperCase();
}
});
return DeepResourceModel;
});
include the new resource model in the manifest
automatic capitalization by the resource model
keys are replaced inside translatable texts
ResourceModel.loadRresourceBundle(..)
. After that point the requested keys are translated by referring to the getText(..)
method of the resource bundle. getText(..)
will take care of matching a key with a translated text.loadResourceBundle
method always instantiates a standard sap/base/i18n/ResourceBundle
class, so we can't insert our own.ResourceBundle.getText(..)
because it is more versatile than the getProperty(..)
method of the resource model. We modify the object (ResourceBundle) returned by the loadResourceBundle method.sap.ui.define([
"sap/ui/model/resource/ResourceModel"
], function(ResourceModel) {
"use strict";
var DeepResourceModel = ResourceModel.extend("com.sample.DeepResourceModel", {
constructor: function (oData) {
oData.DeepResourceModel = this;
ResourceModel.apply(this, arguments);
}
});
const KEYREF = new RegExp(/\(\(\((.*?)\)\)\)/, 'g');
DeepResourceModel.getText = function(sKey, aArgs, bIgnoreKeyFallback) {
let sTemplate = this.bundle.inheritedGetText( sKey, aArgs, bIgnoreKeyFallback );
let sTranslated = sTemplate;
for (const captureGroup of sTemplate.matchAll( KEYREF ) ) {
let sub = this.bundle.getText( captureGroup[1] , [], bIgnoreKeyFallback )
sTranslated = sTranslated.replace( captureGroup[0], sub );
}
return sTranslated
};
ResourceModel.inheritedLoadResourceBundle = ResourceModel.loadResourceBundle;
ResourceModel.loadResourceBundle = async function(oData, bAsync) {
let oRB = ResourceModel.inheritedLoadResourceBundle(oData, bAsync);
if ( bAsync ) {
oRB = await oRB;
}
if ( oData.DeepResourceModel && oRB && !oRB.inheritedGetText ) {
oRB.inheritedGetText = oRB.getText;
oRB.getText = DeepResourceModel
.getText.bind({
DeepResourceModel: oData.DeepResourceModel,
bundle: oRB });
}
return oRB;
}
return DeepResourceModel;
});
DeepResourceBundle
) will be used later to provide a means for the getText
method to access the resource model instance. This will be necessary for more complex scenarios.,loadResourceBundle
of the ResourceModel
. This is a static method so there is no way to overwrite it in descendant classes like our DeepResourceModel
. You can see in the code, that the original function is saved in inheritedLoadResourceBundle
and we will use it to provide the original functionality.ResourceBundle
instance. This means we will not make changes to the base class itself. We only do the hook when we are working with our new DeepResourceModel
. (see the conditional statement). Remember, the loadResourceBundle
is overwritten for all resource models, so even the standard ones will try to use it.getText
and then assign our implementation to it. The important thing to note here is the context we provide for this function. Normally the getText
method would access the resourceBundle itself. However I wanted to give the method a way to access the DeepResourceModel
which was used to create it. Therefore the bind()
function will bind a structure which contains both the ResourceBundle and a reference to the DeepResourceModel.this.bundle.inheritedGetText()
.sTemplate
) it may contain references to other keys. For example: (((attitude)))
. The keys are extracted by a regular expression and each of them is processed. This is where recursion comes handy. We call ourselves but this time only using the key "attitude" to get the results. When the function returns it will contain "lazy" which is than substituted for the key.You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
50 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
2 |