A Brief History
Messages in ABAP as in all other programming languages are used to describe what is happening during the code execution to the programmer or user. They were first invented acting as dialog messages to display short information and handle incorrect user inputs during the classic dynpro processing. Here, an exception situation usually required the direct output of a message to the user. As a good example, one could run the
dynpro input check of ABAP documentation. This shows the oldest way of message instruction in ABAP. It is taken care that the input is given correctly by forcing the user to enter certain values in the fields. Let us take a closer look at a part of this code:
IF input1 < 50.
MESSAGE e888(sabapdemos) WITH text-001 '50' text-002.
ENDIF.
After running the program, by pressing enter, you got a notification in such a form:

This message reminds the user to enter a correct amount and by this, fulfill requirements of messages in the first place.
In early ABAP world, everything used to be dialog programming. However, later messaging concept was adopted for other situations, such as targeted program terminations or handling exceptions.
Before exception classes were introduced, one could handle the error message in a function module as an exception with text via the general exception ‘error_message’. Such non-class-based exceptions can also be handled in function modules as well as class definitions by defining an exception and ‘raising’ it.
Later, it was demanded to associate objects with messages and display them using the statement MESSAGE. This was intended mainly for exception texts of exception classes. As the exception class concept is defined, the main question was, how one can connect the messages to the classes. That was the reason for the emergence of system interfaces IF_T100_MESSAGE and IF_T100_DYN_MSG which can be linked to classes to make classes as carriers of messages. However, this causes complexity due to a double indirection.
IF_T100_MESSAGE and IF_T100_DYN_MSG objects, and in particular exception objects, can also become carriers of any message. This allows "old" exceptions to be turned into new ones. The entire syntax is then only used to get the contents of the system fields that were filled with MESSAGE into the attributes of the classes. In the following, I try to explain it all in more details. So, if you are also curious to know how messaging evolved in ABAP, please accompany me.
Starting with Messages in ABAP
Messages are texts that are created using a message maintenance (transaction SE91) and stored in the system table T100. In ABAP programs, the statement
MESSAGE is the main element for using messages. It is relatively old and invented to handle the dialogs errors. In its basic form, this statement sends a message, by specifying a message type and subsequent program behavior. It is also possible to determine how the message must be shown on the display by defining the display type. Message type is always context sensitive.
For the basic form of the statement MESSAGE, the message type defines how the message is displayed and determines the subsequent program flow. Valid message types are A, E, I, S, W, and X. The abbreviations stand for termination (abort) message, error message, information message, status message, warning, and exit message.
You can mainly add Messages to the program in two ways. Either by hardcoding the message text in the program or by using the message class. To be more precise, the former is like using the message format to send any kind of text in the program. In this case, no definition was required before coding the message in the program.
In case of using message class, messages get displayed from the message class that is defined by using MESSAGE-ID shortly shown as id. Messages number ranges from 000 to 999 (i.e., 1000 messages). Each message associated with message number and the message length can be up to 80 characters.
Originally, one could send a static message using the syntax
…MESSAGE tn(id) …
to specify a message from the database table T100 where t and n are used as the single-character message type and the three-digit message number directly one after another. In the static long form, the message class is specified directly in parentheses using id.
Example I
Display of the short text of the message with the number 001 from the message class ZCL_MSG_DEMO as an information message. First, Let’s create a message class

Now we define message 000
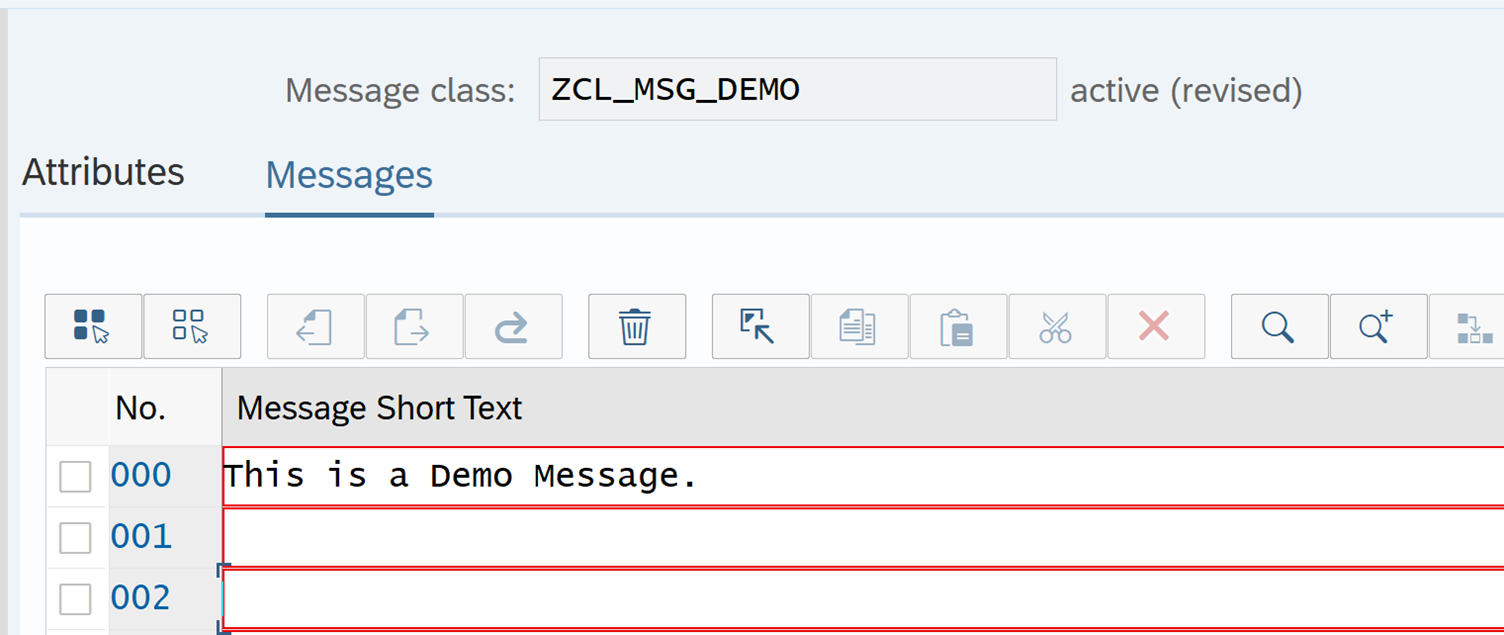
write the code as
MESSAGE i000(ZCL_MSG_DEMO).

You can give the message id once and for all in the beginning of the program. So, the syntax can be even shorter!
REPORT ZBLOG MESSAGE-ID ZCL_MSG_DEMO.
MESSAGE i000.
As said, the actual system behavior after a message is sent, is highly context dependent. The current version of the ABAP keyword documentation contains a
detailed list of effects caused by different message types in different contexts (such as dialog processing, background processing, during an RFC and during the processing of HTTP requests).
For example, in case of list processing, such a message will terminate the program.
REPORT ZBLOG MESSAGE-ID ZCL_MSG_DEMO.
START-OF-SELECTION.
MESSAGE e003.
Also, in list processing, a message of type W is always converted to type E before further context-dependent handling takes place. So, such warnings also terminate the program!
Now, if we change it to a
dialog processing, the behavior is changed depending on the dialog modules or event blocks in which the message is sent:
REPORT zblog MESSAGE-ID zcl_msg_demo.
PARAMETERS p.
AT SELECTION-SCREEN.
IF p IS INITIAL.
MESSAGE e003.
ENDIF.
The Error Message will come up until the condition is fulfilled.
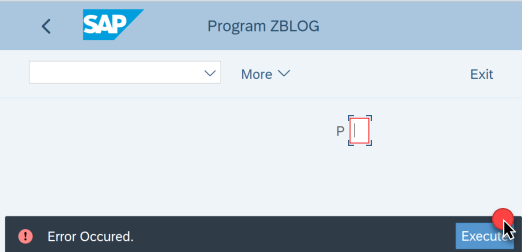
This is how messages intended to control the dialogs. Since there are always other contexts apart from dialog processing, SAP had to invent ways to handle such cases. So, other message types were defined.
Dynamic Form of Messages
Later, the dynamic form of messages is introduced as:
MESSAGE ID mid TYPE mtype NUMBER num.
The message class, the message type, and the message number are specified as content of the data objects mid, mtype, and num. mid and mtype expect character-like data objects that must contain the message class or the message type in uppercase letters. Invalid message types raise an uncatchable exception. num expects a data object of the type n and length 3. In our case they would be:
DATA: mid TYPE sy-msgid VALUE ' ZCL_MSG_DEMO ',
mtype TYPE sy-msgty VALUE 'I',
num TYPE sy-msgno VALUE '000'.
The big difference between dynamic and static form of messaging is that in dynamic form you can use a variable in message statement like
DATA(class) = 'ZCL_MSG_DEMO' .
MESSAGE id class TYPE 'I' NUMBER '001'.
But such thing won’t be possible in the static form.
When a message number used in the program, the corresponding message retrieved from the message class and gets displayed. During this process, information about the exception text is passed to the handler through the system fields. Also, up to four operands can be specified. The MESSAGE...WITH statement used to pass the dynamic or runtime text during the program execution. The optional addition WITH is used to provide the placeholders of a message. The syntax is
MESSAGE ID sy-msgid TYPE sy-msgty NUMBER sy-msgno
WITH sy-msgv1 sy-msgv2 sy-msgv3 sy-msgv4 DISPLAY LIKE dtype.
where
System Fields
Name |
Meaning |
sy-msgid |
Contains the message class after a message is sent. |
sy-msgno |
Contains the message number after a message is sent. |
sy-msgty |
Contains the identifier of the message type with which the message or the text was sent. |
sy-msgv1
to sy-msgv4 |
After a message is sent, contain the content of the data objects specified after the addition WITH in the order of the objects. After any text is sent, contain the first 200 characters of the data object text. |
The position of an operand determines which placeholder is replaced. For example, the formatted content of the operands field1, …, field4 is assigned in sequence to the system fields sy-msgv1 to sy-msgv4. When the addition DISPLAY LIKE is used, the icon of the message type specified in dtype is displayed instead of the associated icon. dtype expects a character-like data objects containing one of the values "A", "E", "I", "S", or "W" in uppercase letters. Please note that the usage of this addition does not influence the behavior determined by the message type, but only the type of display.
Example II
In the defined message class, we create another message with three place holders

Now you can refer to this message as
START-OF-SELECTION.
MESSAGE id 'ZCL_MSG_DEMO' TYPE 'I' NUMBER '001' WITH 'Eat' 'Drink' 'Rest'.
The out put is as expected:

It can also be written in a short form as
MESSAGE i001(ZCL_MSG_DEMO) WITH 'Eat' 'Drink' 'Rest'.
The statement
MESSAGE with the addition
INTO assigns the short text of the message to the target field text. The program flow is not interrupted, and no message processing takes place.
The text can be either an existing character-like variable or an inline declaration DATA(var) or FINAL(var), where a variable of type string is declared. The syntax is
MESSAGE ID sy-msgid TYPE sy-msgty NUMBER sy-msgno
INTO DATA(mtext)
WITH sy-msgv1 sy-msgv2 sy-msgv3 sy-msgv4.
Example III
As of the previous example, the short text of a message is assigned to the data object
mtext declared inline using the corresponding system fields.
MESSAGE ID 'ZCL_MSG_DEMO' TYPE 'I' NUMBER '000'
INTO DATA(mtext)
WITH 'Eat' 'Drink' 'Rest'.
cl_demo_output=>display( mtext ).
As said, one can use message syntax to simply send a text out. The syntax for issuing such messages is as follows.
MESSAGE <message> TYPE <message type>.
- Message- 80 characters user defined message.
- Message-type- Specifies the message type.
Example IV
The message coding is simple and as follows
* Displaying error Message of type E
MESSAGE 'This is an Error message' TYPE 'E'.
Which shows as

The usage of messages can vary in the different programs and functional requirements. The programmer should take care of a proper and good professional usage of messaging.
Summary
Here, I explained the basics of messaging in ABAP. in the
next post, I will introduce (non)class-based exceptions and exception classes that include message interfaces in more details. So, stay tuned!