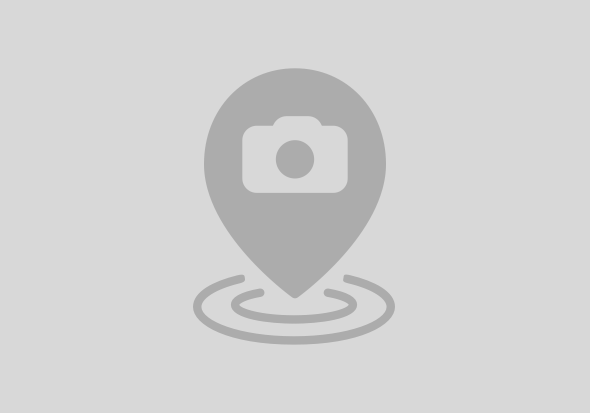
using {API_BUSINESS_PARTNER as external} from './external/API_BUSINESS_PARTNER.csn';
service AddressManagerService {
@readonly
entity BusinessPartners as projection on external.A_BusinessPartner {
BusinessPartner, LastName, FirstName, to_BusinessPartnerAddress
};
entity BusinessPartnerAddresses as projection on external.A_BusinessPartnerAddress {
BusinessPartner, AddressID, Country, PostalCode, CityName, StreetName, HouseNumber
}
};
BusinessPartner;LastName;FirstName
30413010;Smith;John
30413011;Thomson;Georg
"credentials": {
"url": "https://sandbox.api.sap.com/s4hanacloud/sap/opu/odata/sap/API_BUSINESS_PARTNER/"
}
const cds = require('@sap/cds');
//here is the service implementation
//here are the service handlers
module.exports = cds.service.impl(async function () {
//these are the entities from address-manager-service.cds file
const { BusinessPartners, BusinessPartnerAddresses } = this.entities;
//cds will connect to the external service API_BUSINESS_PARTNER
//which is declared in package.json in the cds requires section
const service = await cds.connect.to('API_BUSINESS_PARTNER');
//this event handler is triggered when we call
//GET http://localhost:4004/address-manager/BusinessPartners
this.on('READ', BusinessPartners, async (req) => {
try {
const tx = service.transaction();
//entity name as it is in the .csn file for the service API_BUSINESS_PARTNER
let entity = 'A_BusinessPartner';
//columns which we have declared in cds entity that we want to expose
let columnsToSelect = ["BusinessPartner", "FirstName", "LastName"];
return await tx.emit({
query: SELECT.from(entity)
.columns(columnsToSelect),
//For API Business Hub usage, we send custom APIKey header
headers: {
"APIKey": process.env.S4_APIKEY
}
})
} catch (err) {
req.reject(err);
}
});
//this event handler is triggered when we call
//GET http://localhost:4004/address-manager/BusinessPartnerAddresses
this.on('READ', BusinessPartnerAddresses, async (req) => {
try {
const tx = service.transaction();
//entity name as it is in the .csn file for the service API_BUSINESS_PARTNER
let entity = 'A_BusinessPartnerAddress';
//columns which we have declared in cds entity that we want to expose
let columnsToSelect = ["BusinessPartner", "AddressID", "Country", "PostalCode", "CityName", "StreetName", "HouseNumber"];
return await tx.emit({
query: SELECT.from(entity)
.columns(columnsToSelect),
//For API Business Hub usage, we send custom APIKey header
headers: {
"APIKey": process.env.S4_APIKEY
}
})
} catch (err) {
req.reject(err);
}
});
});
$env:S4_APIKEY=">>>YOUR API KEY<<<"
$env:S4_APIKEY="8JLQURhRAeEH8OHgXFTXcfmW6H0vrW5V”
"credentials": {
"destination": "s4hc"
}
const cds = require('@sap/cds');
//here is the service implementation
//here are the service handlers
module.exports = cds.service.impl(async function () {
//these are the entities from address-manager-service.cds file
const { BusinessPartners, BusinessPartnerAddresses } = this.entities;
//cds will connect to the external service API_BUSINESS_PARTNER
//which is declared in package.json in the cds requires section
const service = await cds.connect.to('API_BUSINESS_PARTNER');
//this event handler is triggered when we call
//GET http://localhost:4004/address-manager/BusinessPartners
this.on('READ', BusinessPartners, async (req) => {
try {
const tx = service.transaction();
//entity name as it is in the .csn file for the service API_BUSINESS_PARTNER
let entity = 'A_BusinessPartner';
//columns which we have declared in cds entity that we want to expose
let columnsToSelect = ["BusinessPartner", "FirstName", "LastName"];
return await tx.run(
SELECT.from(entity)
.columns(columnsToSelect)
)
} catch (err) {
req.reject(err);
}
});
//this event handler is triggered when we call
//GET http://localhost:4004/address-manager/BusinessPartnerAddresses
this.on('READ', BusinessPartnerAddresses, async (req) => {
try {
const tx = service.transaction();
//entity name as it is in the .csn file for the service API_BUSINESS_PARTNER
let entity = 'A_BusinessPartnerAddress';
//columns which we have declared in cds entity that we want to expose
let columnsToSelect = ["BusinessPartner", "AddressID", "Country", "PostalCode", "CityName", "StreetName", "HouseNumber"];
return await tx.run(
SELECT.from(entity)
.columns(columnsToSelect)
)
} catch (err) {
req.reject(err);
}
});
});
requires:
- name: uaa
- name: dest
resources:
- name: uaa
type: org.cloudfoundry.managed-service
parameters:
path: ./xs-security.json
service: xsuaa
service-name: my-uaa
service-plan: application
- name: dest
type: org.cloudfoundry.managed-service
parameters:
service: destination
service-name: my-dest
service-plan: lite
"build": "mbt build -p=cf --mtar=AddressManager.mtar",
"deploy": "cf deploy mta_archives/AddressManager.mtar"
{
"VCAP_SERVICES": {…},
"VCAP_APPLICATION": {…}
}
"watch": "npx cds watch"
{
"command": "cds watch",
"name": "cds watch",
"request": "launch",
"type": "node-terminal",
"skipFiles": [
"<node_internals>/**"
]
}
const proxy = require('@sap/cds-odata-v2-adapter-proxy')
const cds = require('@sap/cds')
cds.on('bootstrap', app => app.use(proxy()))
module.exports = cds.server
const cds = require('@sap/cds');
//here is the service implementation
//here are the service handlers
module.exports = cds.service.impl(async function () {
//these are the entities from address-manager-service.cds file
const { BusinessPartners, BusinessPartnerAddresses } = this.entities;
//cds will connect to the external service API_BUSINESS_PARTNER
//which is declared in package.json in the cds requires section
const service = await cds.connect.to('API_BUSINESS_PARTNER');
//this event handler is triggered when we call
//GET http://localhost:4004/address-manager/BusinessPartners
this.on('READ', BusinessPartners, async (req) => {
try {
const tx = service.transaction();
//entity name as it is in the .csn file for the service API_BUSINESS_PARTNER
let entity = 'A_BusinessPartner';
//columns which we have declared in cds entity that we want to expose
let columnsToSelect = ["BusinessPartner", "FirstName", "LastName"];
//if there is a parameter
if (req.params[0]) {
//If you look in the .csn file you will see that
//the key for our BusinessPartner entity is BusinessPartner column
const businessPartner = req.params[0].BusinessPartner;
return await tx.run(
SELECT.from(entity)
.columns(columnsToSelect)
.where({ 'BusinessPartner': businessPartner })
)
} else {
//if no parameter, we read all Business Partners
return await tx.run(
SELECT.from(entity)
.columns(columnsToSelect)
)
}
} catch (err) {
req.reject(err);
}
});
//this event handler is triggered when we call
//GET http://localhost:4004/address-manager/BusinessPartnerAddresses
this.on('READ', BusinessPartnerAddresses, async (req) => {
try {
const tx = service.transaction();
//entity name as it is in the .csn file for the service API_BUSINESS_PARTNER
let entity = 'A_BusinessPartnerAddress';
//columns which we have declared in cds entity that we want to expose
let columnsToSelect = ["BusinessPartner", "AddressID", "Country", "PostalCode", "CityName", "StreetName", "HouseNumber"];
//if there is parameter
if (req.params[0]) {
//If you look in the .csn file you will see that
//the keys for our BusinessPartnerAddress entity are
//BusinessPartner and AddressID columns
const businessPartner = req.params[0].BusinessPartner;
const addressId = req.params[0].AddressID;
return await tx.run(
SELECT.from(entity)
.columns(columnsToSelect)
.where({
'BusinessPartner': businessPartner,
'AddressID': addressId
})
)
} else {
//if no parameter, we read all Business Partner Addresses
return await tx.run(
SELECT.from(entity)
.columns(columnsToSelect)
)
}
} catch (err) {
req.reject(err);
}
});
});
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
43 | |
25 | |
17 | |
15 | |
10 | |
7 | |
7 | |
6 | |
6 | |
6 |