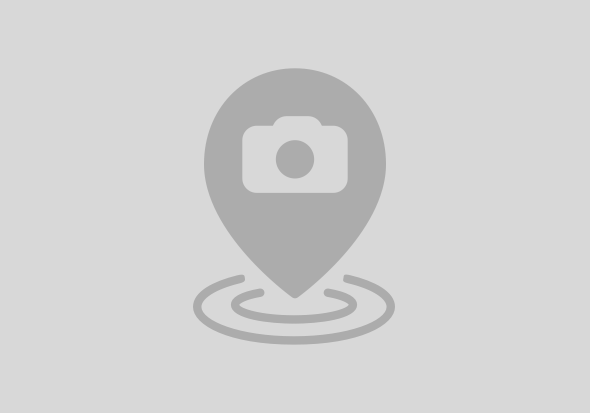
For this demo, we will require the following SAP frameworks.
import SwiftUI
import SAPFiori
struct ObjectTableView {
// MARK: - Properties
typealias UIViewType = UITableView
let tableView = UITableView(frame: .zero, style: .grouped)
let objectCellId = "TableCellIdentifier"
}
// MARK: - UIViewRepresentable
extension ObjectTableView: UIViewRepresentable {
func makeUIView(context: Context) -> UITableView {
setupDatasourceDelegate(context)
setupTable()
return tableView
}
func updateUIView(_ uiView: UITableView, context: Context) { }
func makeCoordinator() -> ObjectTableViewCoordinator {
ObjectTableViewCoordinator(self)
}
}
// MARK: - Private
private extension ObjectTableView {
func setupTable() {
tableView.estimatedRowHeight = 80
tableView.rowHeight = UITableView.automaticDimension
tableView.register(FUIObjectTableViewCell.self, forCellReuseIdentifier: objectCellId)
tableView.tableHeaderView = profileHeader
}
func setupDatasourceDelegate(_ context: Context) {
tableView.dataSource = context.coordinator
tableView.delegate = context.coordinator
}
var profileHeader: FUIProfileHeader {
let profileHeader = FUIProfileHeader()
profileHeader.imageView.image = UIImage(named: "User") // Replace image
profileHeader.headlineText = "Headline"
profileHeader.subheadlineText = "Subheadline"
profileHeader.descriptionText = "SAP Fiori iOS component integration with SwiftUI."
let activityControl = FUIActivityControl()
activityControl.addActivities([.phone, .message, .email])
activityControl.activityItems[.phone]?.tintColor = UIColor.preferredFioriColor(forStyle: .primary6)
activityControl.activityItems[.message]?.tintColor = UIColor.preferredFioriColor(forStyle: .primary6)
activityControl.activityItems[.email]?.tintColor = UIColor.preferredFioriColor(forStyle: .primary6)
profileHeader.detailContentView = activityControl
return profileHeader
}
}
// MARK: - Coordinator
class ObjectTableViewCoordinator: NSObject {
// MARK: - Properties
var parent: ObjectTableView
// MARK: - Initialization
init(_ parent: ObjectTableView) {
self.parent = parent
}
}
// MARK: - UITableViewDataSource, UITableViewDelegate
extension ObjectTableViewCoordinator: UITableViewDataSource, UITableViewDelegate {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 5
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let objectCell = tableView.dequeueReusableCell(withIdentifier: parent.objectCellId,
for: indexPath as IndexPath) as! FUIObjectTableViewCell
objectCell.headlineText = "Headline Text"
objectCell.subheadlineText = "Subheadline Text"
objectCell.footnoteText = "Footer Note Text"
objectCell.descriptionText = "SAP Fiori iOS component integration with SwiftUI."
objectCell.detailImage = UIImage(named: "SwiftUI") // Replace it with your image
objectCell.substatusText = "In Stock"
objectCell.substatusLabel.textColor = .preferredFioriColor(forStyle: .positive)
objectCell.accessoryType = .disclosureIndicator
objectCell.splitPercent = CGFloat(0.3)
return objectCell
}
}
import SwiftUI
struct ContentView: View {
// MARK: - Body
var body: some View {
NavigationView {
ObjectTableView()
.navigationBarTitle("Swift UI Fiori Demo", displayMode: .inline)
}
.navigationViewStyle(StackNavigationViewStyle())
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
iPad
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
14 | |
10 | |
8 | |
6 | |
6 | |
6 | |
6 | |
6 | |
5 | |
4 |