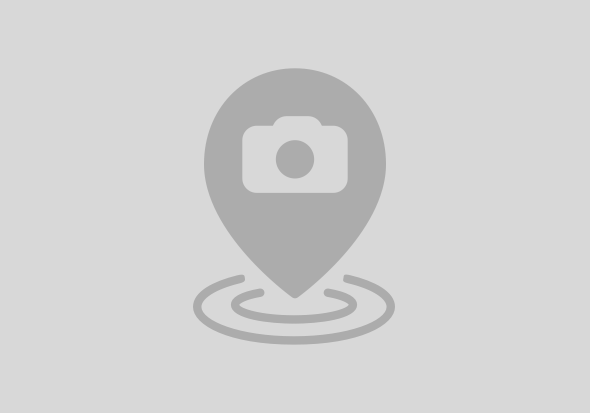
using {sap} from '@sap/cds/common';
namespace covid19;
entity CountriesAggregated {
key Date : Date;
key Country : String;
Confirmed : Integer;
Recovered : Integer;
Deaths : Integer;
}
using {covid19} from '../db/schema';
service Covid19Service {
@readonly
entity CountriesAggregated as projection on covid19.CountriesAggregated;
}
const path = require('path')
const fs = require('fs-extra')
const stream = require('stream')
const util = require('util')
const finished = util.promisify(stream.finished)
const changeCase = require('change-case')
const { Dataset } = require('data.js')
const endpoint = 'https://datahub.io/core/covid-19/datapackage.json'
const names = ['countries-aggregated']
module.exports = (grunt) => async function () {
const done = this.async()
try {
// ensure csv dir
const dirpath = path.join(process.cwd(), 'db', 'data')
await fs.emptyDir(dirpath)
// load dataset
const dataset = await Dataset.load(endpoint)
// get all tabular data (if exists any)
for (const id in dataset.resources) {
const resource = dataset.resources[id]
const { name, format } = resource._descriptor
// filter resources
if (format === 'csv' && names.includes(name)) {
// write resource to .csv file
const readable = await resource.stream()
const filepath = path.join(dirpath, `covid19-${changeCase.pascalCase(name)}.csv`)
const writable = fs.createWriteStream(filepath)
readable.pipe(writable)
await finished(writable)
}
}
done()
} catch (error) {
grunt.log.error(error.stack)
done(error)
}
}
{
"scripts": {
"prestart": "npx grunt && npx cds deploy",
"start": "npx cds run"
}
npm start
http://localhost:4004/covid19/CountriesAggregated?
$filter=Country eq 'Germany'"
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
5 | |
3 | |
3 | |
2 | |
2 | |
2 | |
2 | |
2 | |
1 | |
1 |