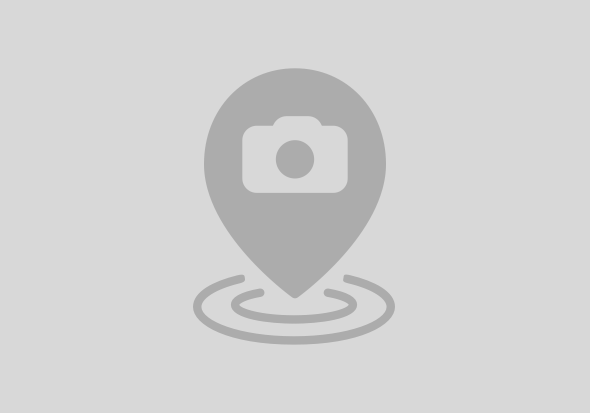
ID: sap-cf-axios-example
_schema-version: "2.1"
description: Example sap cf axios
version: 0.0.1
modules:
- name: sca_approuter
type: approuter.nodejs
path: approuter
parameters:
disk-quota: 256M
memory: 256M
requires:
- name: sca_uaa
- name: sca_destination_service
- name: sca_api
group: destinations
properties:
forwardAuthToken: true
name: sca_api
strictSSL: false
url: '~{url}'
- name: sca_srv
type: nodejs
path: srv
parameters:
memory: 512M
disk-quota: 256M
provides:
- name: sca_api
properties:
url: ${default-url}
requires:
- name: sca_uaa
- name: sca_connectivity_service
- name: sca_destination_service
resources:
- name: sca_destination_service
type: destination
parameters:
service-plan: lite
shared: true
- name: sca_connectivity_service
type: connectivity
parameters:
service-plan: lite
shared: true
- name: sca_uaa
type: com.sap.xs.uaa
parameters:
service: xsuaa
service-plan: application
path: ./xs-security.json
const axios = require('axios');
const response = await axios({
method: "post",
url: "/BookSet",
baseUrl: "https://localhost:3002"
data: {
title: "Using Axios in SAP Cloud Foundry",
author: "Joachim Van Praet"
},
headers: {
"content-type": "application/json"
},
auth: {
username: 'jowa',
password: 's00pers3cret'
}
})
npm install -s sap-cf-axios
const {SapCfAxios} = require('sap-cf-axios');
const destinationName = "MyDestinationNameInCloudFoundry";
const axios = SapCfAxios(destinationName);
const response = await axios({
method: "post",
url: "/BookSet",
data: {
title: "Using Axios in SAP Cloud Foundry",
author: "Joachim Van Praet"
},
headers: {
"content-type": "application/json"
}
})
npm install
npm run start
POST /BookSet HTTP/1.1
Host: localhost:3002
Content-Type: application/json
Authorization: Basic am93YTpzMDBwZXJzM2NyZXQ=
User-Agent: PostmanRuntime/7.19.0
Accept: */*
Cache-Control: no-cache
Postman-Token: ab328757-526e-4ab3-9464-d454a9abd9a1,157664fa-0efa-41ea-a97a-f440e836eb81
Host: localhost:3002
Accept-Encoding: gzip, deflate
Content-Length: 89
Connection: keep-alive
cache-control: no-cache
{
"title": "Using Axios in SAP Cloud Foundry",
"author": "Joachim Van Praet"
}
{
"method": "POST",
"baseUrl": "",
"uri": "/BookSet",
"host": "localhost",
"headers": {
"content-type": "application/json",
"authorization": "Basic am93YTpzMDBwZXJzM2NyZXQ=",
"user-agent": "PostmanRuntime/7.19.0",
"accept": "*/*",
"cache-control": "no-cache",
"postman-token": "ab328757-526e-4ab3-9464-d454a9abd9a1",
"host": "localhost:3002",
"accept-encoding": "gzip, deflate",
"content-length": "89",
"connection": "keep-alive"
},
"body": {
"title": "Using Axios in SAP Cloud Foundry",
"author": "Joachim Van Praet"
}
}
const express = require('express');
const app = express();
const {SapCfAxios} = require('sap-cf-axios');
// the destination that we want to use is named 'DUMMY'
const axios = SapCfAxios('DUMMY');
app.use(express.json());
const handleRequest = async (req, res) => {
try {
const response = await axios({
method: 'POST',
url: '/BookSet',
data: {
title: "Using Axios in SAP Cloud Foundry",
author: "Joachim Van Praet"
},
headers: {
"content-type": "application/json"
/* if you are using an oauth2* authentication type or principal propagation
* for your destination
* please add your JWT token in the authorization header of this request
**/
// authorization: <user JWT Token>
}
});
res.send(response.data);
} catch (error) {
res.reject(error);
}
}
app.all('*', handleRequest);
const port = process.env.PORT || 3000;;
app.listen(port, function () {
console.log('myapp listening on port ' + port);
});
npm install
npm run build
npm run deploy
{
"method": "POST",
"baseUrl": "",
"uri": "/BookSet",
"host": "localhost",
"headers": {
"accept": "application/json, text/plain, */*",
"content-type": "application/json",
"authorization": "Basic am9hY2hpbTpzMDBwZXJzM2NyZXQ=",
"sap-connectivity-scc-location_id": "my_cloud_connector_location_id",
"user-agent": "axios/0.19.0",
"content-length": "73",
"connection": "close",
"host": "localhost:3002",
"x-forwarded-host": "dummy:3000"
},
"body": {
"title": "Using Axios in SAP Cloud Foundry",
"author": "Joachim Van Praet"
}
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
54 | |
5 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |