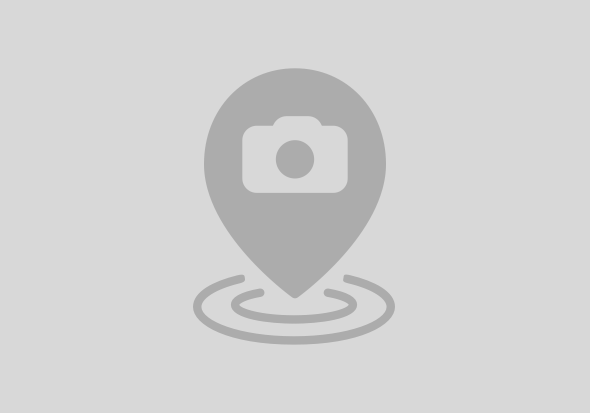
METHOD IF_HTTP_EXTENSION~HANDLE_REQUEST.
***************************************************************************
" VARIABLES
***************************************************************************
DATA: LO_REST_CLASS TYPE REF TO ZIF_REST.
DATA: LO_ERROR TYPE REF TO CX_ROOT.
DATA: LV_REASON TYPE STRING.
***************************************************************************
" GET THE CLASS OBJECT
***************************************************************************
TRY.
LO_REST_CLASS ?= GET_REST( IO_SERVER = SERVER ).
***************************************************************************
" EXECUTE THE RETRIEVED CLASS
***************************************************************************
LO_REST_CLASS->HANDLE_REQUEST( ).
***************************************************************************
" ERROR
***************************************************************************
CATCH CX_ROOT INTO LO_ERROR.
LV_REASON = LO_ERROR->GET_TEXT( ).
SERVER->RESPONSE->SET_STATUS( CODE = 500
REASON = LV_REASON ).
ENDTRY.
ENDMETHOD.
METHOD GET_REST.
***************************************************************************
" VARIABLES
***************************************************************************
DATA: LV_CLASS_NAME TYPE SEOCLSNAME.
DATA: LV_REQUEST_METHOD TYPE STRING.
***************************************************************************
" APPEND REQUEST METHOD TO BASE CLASS
***************************************************************************
LV_REQUEST_METHOD = IO_SERVER->REQUEST->GET_HEADER_FIELD( '~request_method' ).
CONCATENATE 'ZCL_REST_TEST_' LV_REQUEST_METHOD INTO LV_CLASS_NAME.
***************************************************************************
" RETURN CLASS OBJECT
***************************************************************************
TRY.
CREATE OBJECT EO_REST
TYPE (LV_CLASS_NAME)
EXPORTING
IO_REQUEST = IO_SERVER->REQUEST
IO_RESPONSE = IO_SERVER->RESPONSE.
***************************************************************************
" ERRORS
***************************************************************************
CATCH CX_SY_CREATE_OBJECT_ERROR.
ENDTRY.
ENDMETHOD.
METHOD ZIF_REST~HANDLE_REQUEST.
***************************************************************************
" VARIABLES
***************************************************************************
DATA: LT_EQUIPS TYPE ZTT_TEST_EQUI.
DATA: LV_STRING_WRITER TYPE REF TO CL_SXML_STRING_WRITER.
DATA: LV_XSTRING TYPE XSTRING.
***************************************************************************
" EXECUTE GET_EQUIPMENTS METHOD
***************************************************************************
TRY.
LT_EQUIPS = GET_EQUIPMENTS( ME->ZIF_REST~REQUEST ).
***************************************************************************
" CONVERT EQUIPMENTS TO JSON
***************************************************************************
LV_STRING_WRITER = CL_SXML_STRING_WRITER=>CREATE( TYPE = IF_SXML=>CO_XT_JSON ).
CALL TRANSFORMATION ID SOURCE ARRAY = LT_EQUIPS RESULT XML LV_STRING_WRITER.
LV_XSTRING = LV_STRING_WRITER->GET_OUTPUT( ).
***************************************************************************
" ADD THE JSON EQUIPMENTS TO THE RESPONSE
***************************************************************************
ME->ZIF_REST~RESPONSE->SET_DATA( DATA = LV_XSTRING ).
CATCH CX_ROOT.
ENDTRY.
ENDMETHOD.
METHOD ZIF_REST~SET_RESPONSE.
CALL METHOD ME->ZIF_REST~RESPONSE->SET_DATA
EXPORTING
DATA = IS_DATA.
ENDMETHOD.
METHOD CONSTRUCTOR.
ME->ZIF_REST~RESPONSE = IO_RESPONSE.
ME->ZIF_REST~REQUEST = IO_REQUEST.
ENDMETHOD.
METHOD GET_EQUIPMENTS.
***************************************************************************
" VARIABLES
***************************************************************************
DATA: LV_EQUI_NUMBER TYPE EQUNR.
***************************************************************************
" GET HEADER PARAMETERS VALUE FROM URL
***************************************************************************
LV_EQUI_NUMBER = ME->ZIF_REST~REQUEST->GET_FORM_FIELD('equnr').
UNPACK LV_EQUI_NUMBER TO LV_EQUI_NUMBER.
***************************************************************************
" GET EQUIPMENTS SELECT
***************************************************************************
SELECT EQUI~EQUNR, EQKT~EQKTX
FROM EQUI AS EQUI
LEFT OUTER JOIN EQKT AS EQKT ON EQKT~EQUNR EQ EQUI~EQUNR
INTO TABLE @ET_EQUIPMENTS
WHERE EQUI~EQUNR EQ @LV_EQUI_NUMBER
AND EQKT~SPRAS EQ 'E'.
ENDMETHOD.
METHOD ZIF_REST~HANDLE_REQUEST.
***************************************************************************
" TYPES
***************************************************************************
TYPES: BEGIN OF TYPE_EQUI,
EQUNR TYPE STRING,
EQKTX TYPE STRING,
END OF TYPE_EQUI.
***************************************************************************
" VARIABLES AND OBJECTS
***************************************************************************
DATA: LS_EQUI TYPE TYPE_EQUI.
DATA: LT_EQUIS TYPE TABLE OF TYPE_EQUI.
DATA: LR_DESERIALIZER TYPE REF TO CL_TREX_JSON_DESERIALIZER.
DATA: LV_JSON_BODY TYPE STRING.
DATA: LV_STRING_WRITER TYPE REF TO CL_SXML_STRING_WRITER.
DATA: LV_XSTRING TYPE XSTRING.
CREATE OBJECT LR_DESERIALIZER.
***************************************************************************
" JSON TO ABAP DATA
***************************************************************************
LV_JSON_BODY = ME->ZIF_REST~REQUEST->GET_CDATA( ).
LR_JSON_DESERIALIZER->DESERIALIZE(
EXPORTING
JSON = LV_JSON_BODY
IMPORTING
ABAP = LS_EQUI ).
APPEND LS_EQUI TO LT_EQUIS.
***************************************************************************
" CREATE OBJECT
***************************************************************************
"DO WHATEVER YOU NEED TO DO HERE WITH THE DATA !!!
***************************************************************************
" CONVERT INPUT TO JSON STRING
***************************************************************************
LV_STRING_WRITER = CL_SXML_STRING_WRITER=>CREATE( TYPE = IF_SXML=>CO_XT_JSON ).
CALL TRANSFORMATION ID SOURCE ARRAY = LT_EQUIS RESULT XML LV_STRING_WRITER.
LV_XSTRING = LV_STRING_WRITER->GET_OUTPUT( ).
***************************************************************************
" RETURN CREATED OBJECT AS RESPONSE (CONVENTION)
***************************************************************************
ME->ZIF_REST~RESPONSE->SET_DATA( DATA = LV_XSTRING ).
ENDMETHOD.
METHOD ZIF_REST~SET_RESPONSE.
CALL METHOD ME->ZIF_REST~RESPONSE->SET_DATA
EXPORTING
DATA = IS_DATA.
ENDMETHOD.
METHOD CONSTRUCTOR.
ME->ZIF_REST~RESPONSE = IO_RESPONSE.
ME->ZIF_REST~REQUEST = IO_REQUEST.
ENDMETHOD.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |