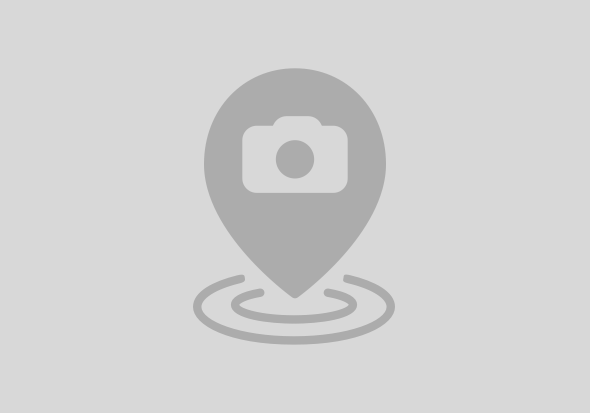
Introduction
This is the third part of my blog series about the basics of UI5 development. The first two parts are about building and theming a website. This was achieved by using HTML and CSS. This blog concentrates on the interaction between the website and the user. This will be done using JavaScript, a script language that descended from C. It enables the webmaster to offer interaction to the user (for example triggering events).
Since this blog series is targeted at veteran ABAP developers I want to ease your transition by comparing ABAP to JavaScript to teach you the basic syntax. After that you can turn to advanced literature to deepen your skills.
JavaScript is essential to developing applications with SAP UI5. Similar to CSS the JavaScript code will be embedded into your website. It is placed as you have learned in part 2 of the blog series in the HTMl tag <head>. However you can insert your JavaScript file everywhere in its own HTML5 tag.
Data types
Contrary to the development in SAP and ABAP Javascript does not have a Data Dictionary to create data elements, structure and tables. It is very loosely typed. There are the following data types:
• Boolean
• Number
• String
• Objects
In ABAP there are four char-like data types alone:
• Char ( C )
• Numchar (N)
• Date (D)
• Timestamp (T)
Declaring variables
The declaration of variables is easier compared to ABAP, where the declaration begins with DATA and a concrete data type or element. For example:
DATA lv_sales_order TYPE VBAK-VBELN.
JavaScript declares by allocation and it uses the keyword "var" instead of "data". For example:
var text = "I am a string";
This declares the variable "text" and allocates the string "I am a string". Instead of the "."-notation in ABAP the statements in JavaScript end with ";".
You may wonder what would happen if you just wrote "var text;". This would declare the variable text but it would have the state "undefined". This can be compared to INITIAL in ABAP. INITIAL does not exist in JavaScript. There are the states "undefined", "not defined", "NaN" (not a number) and "null". "null" is only relevant to objects.
Objectorientation and dot-notation
JavaScript is object oriented and the declaration of objects is easy:
var dateObj = new Date();
In this example I created a date object with the name "dateObj". This can be referenced by the dot-notation to execute ist class methods.
Outputting the current weekday as an example:
var dateObj = new Date( );
var day = dateObj.getDay();
The variable day holds the current weekday now as a number. Monday would be a 1, Tuesday a 2 and so on.
In ABAP an object has to be declared first with "TYPE REF TO". To instantiate an object you use the command "CREATE OBJECT" and to execute a methode you use the "->" arrow.
Im ABAP muss das Objekt zuvor deklariert werden. Hierfür dient die Syntax „TYPE REF TO“. Um ein Objekt zu instanziieren dient der Befehl „CREATE OBJECT“ und um eine Methode aufzurufen wird eine „->“ – Pfeilnotation genutzt.
Example:
DATA lo_date_object TYPE REF TO ZCL_DATE_INFORMATION.
DATA lv_day TYPE i.
CREATE OBJECT lo_date_object.
*return day as number
lv_day = lo_date_object->getDay( ).
Functions
Here's a greate advantage of JavaScript: functions. They can be created fast and easy and can be used directly on the website. There doesn't need to be a return-paramenter and the user can embed the function everywhere on the website:
Example function:
function addNumbers( num1 , num2 ){
alert( num1 + num2 );
}
This simple function returns the sum of "num1" and "num2" using the alert-function. This creates a popup in the browser.
As you can see the curly brackets are used to define a function block. Inside of this block you can create as many local variables as you want.
If you want to use local data in a SAP report you used internal tables. They use a row type or structure. A short example:
DATA: lt_interal_table_of_sales_orders TYPE TABLE OF VBAK.
DATA: ls_line_structure_of_sales_order TYPE VBAK.
With these commands we created an internal table of the DDIC table VBAK and a structure to read rows from this table.
To read data from the internal table you can use a LOOP or READ TABLE.
In this case we want to read the first line of the table:
READ TABLE lt_internal_table_of_sales_orders INTO ls_line_structure_of_sales_order INDEX 1.
Arrays
JavaScript uses arrays, a data structure that is very similar to internal tables in ABAP. They contain a set of elements, which don't need to be of the same type. The index starts at 0 and inserting, updating or deleting of elements is always possible. Creating an array can be compard to instantiating an object.
var exampleArray = new Array( );
You can directly insert values, too:
var exampleArrayWithValues = new Array( „Value1″ , „Value2″, „Value3″);
To read the second value type this:
var a = exampleArrayWithValues[ 1 ];
Variable "a" will now contain the string "Value2". You can also create the array using only the literals:
var exampleArray2 = [ „Value1″, „Value2″ , „Value3″ ];
And that is about it. You don't need to know more to start using JavaScript, a must-have in the toolbox of an UI5 developer.
A first example
In this little example you will create a button with HTML which defines an event onClick (trigged by a click). This event will call the function "buttonClicked".
<html>
<head>
<title>Button-JavaScript</title>
<script type="text/javascript">
function buttonClicked(){
alert("I was clicked!");
}
</script>
</head>
<body>
<button onclick="buttonClicked()">Click me</button>
</body>
</html>
As mentioned before the JavaScript code will be placed inside the header. The function "buttonClicked" does nothing more that creating an alter-popup with the text "I was clicked!". The body of the page contains a button that calls the function "buttonClicked" if the event onClick occurs. And that's it. You created your first interaction between website and user.
Here you can see the website in action:
I hope that I could give you a short overview over the basics of SAP UI5. Maybe I could pique your curiosity and you will start learning more about these technologies. If you have any questions or feedback feel free to contact me.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
2 |